Understanding Multiple Query Parameters in URL Design A 2024 Guide to RESTful API Best Practices
Understanding Multiple Query Parameters in URL Design A 2024 Guide to RESTful API Best Practices - Understanding URL Query Parameter Syntax With Multiple Variable Types
When designing APIs, understanding how to use query parameters effectively, especially when dealing with varied data types, is key. They're those extra bits of information tacked onto the end of a URL after a question mark, formatted as key-value pairs. These can be used for filtering results, sorting data, and customizing API requests, making them a powerful tool for client-side interaction.
However, their power comes with a need for care. You have to be precise about how you encode different data types, especially when a single parameter might need to handle multiple values. A carelessly constructed query parameter can easily lead to API mishaps.
The goal with any API design, including the use of query parameters, should be a balance. You want APIs to be flexible, allowing users to easily customize their requests, but also readable and simple to understand. A URL cluttered with an overwhelming amount of query parameters isn't a good user experience. Keeping them concise and well-structured is crucial for preventing a cluttered mess while still providing the desired customization. It's about finding that sweet spot between providing a flexible, powerful API and one that's still easy to use and maintain.
1. URLs can carry a diverse set of data types within their query parameters, encompassing text, numbers, and true/false values. This flexibility in data transmission aligns with the established URL standards (RFC 3986), though it can also introduce some complexity.
2. Proper encoding is essential when crafting query parameters, as certain symbols can cause problems during transmission. For example, a space needs to be changed to `%20` and `&` to `%26`. Getting this wrong can cause the data to be misinterpreted by the receiving system.
3. While there's no hard limit on a URL's length, it's widely acknowledged that browsers have limitations (typically around 2000 characters). If you have a URL with many parameters, or with very long values for parameters, you could easily run into truncation problems, so be aware of this.
4. The order of query parameters doesn't generally affect how the API handles them, but it certainly can influence how a human understands the URL. A poorly organized string of parameters makes a URL look messy, and while it won't stop it from working, it does raise questions about maintainability and readability.
5. Utilizing URL templates with slots for query parameters can improve API documentation quite a bit. This makes it easier for other developers to understand the intended use of parameters. It becomes a more straightforward experience trying to figure out what API calls look like.
6. It's important to recognize that back-end systems and the frameworks used to build them often come with limitations about the number and type of query parameters they can handle. This varies quite a bit between systems and can cause major headaches if you aren't aware of these limits during development.
7. Browser and content delivery network (CDN) caches sometimes treat query parameters differently unless explicitly told to behave otherwise. How this plays out with an API can cause problems with performance if you haven't considered how it impacts your API's design.
8. If query parameters are not carefully processed, there can be security risks such as SQL injection or XSS vulnerabilities. Implementing robust validation procedures for incoming data is crucial to prevent exploitation through these loopholes.
9. Different programming languages and their libraries have varying defaults when it comes to encoding and decoding URLs. Without careful attention, this can lead to data being interpreted differently across different systems. This is particularly relevant when developing APIs intended for a wider range of environments and clients.
10. Choosing descriptive and meaningful parameter names is a good practice. This not only improves the overall understanding of a request but can also boost visibility on search engines, as the parameters give more context to what resources are being accessed.
Understanding Multiple Query Parameters in URL Design A 2024 Guide to RESTful API Best Practices - Building Resource Based Request Paths Rather Than Parameter Heavy URLs
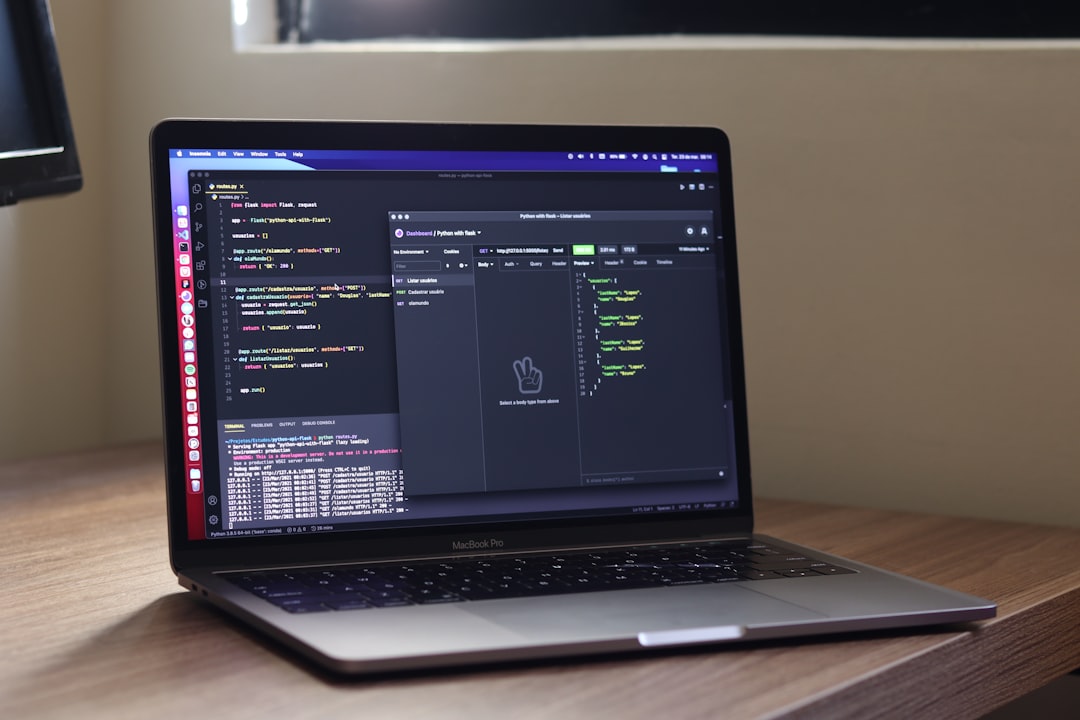
When crafting APIs, it's beneficial to prioritize structuring requests around resources rather than relying heavily on numerous query parameters within URLs. This means designing API endpoints that incorporate path parameters to identify specific resources. This approach avoids creating URLs that become cluttered and difficult to understand. While query parameters are valuable for filtering, sorting, and other request refinements, overusing them can lead to messy, hard-to-read URLs.
By making resource identification the core of your API design, you create a more intuitive and maintainable system. This not only simplifies requests but also makes them easier to understand and implement. The goal is to find a balance where query parameters enhance the flexibility of the API without sacrificing the simplicity and usability of the URLs themselves. This results in a more efficient and user-friendly API experience overall.
1. **Focusing on Resources, Not Just Parameters:** Designing APIs with a focus on resources, like `/users/123/posts`, aligns better with RESTful ideas, making it more obvious how resources are connected. It's a move away from URLs crammed with lots of parameters, which can make the intended resource structure hard to see.
2. **Making URLs Easier to Understand:** URLs structured around resources are simpler for developers and users to grasp, making it more likely they'll use the API correctly. Clear paths mean less mental effort, leading to more self-explanatory API endpoints.
3. **Better Caching Performance:** Resource-based paths can improve caching on both the client and server. This approach allows browsers and content delivery networks to cache responses more effectively when compared to URLs with lots of varying parameters, possibly leading to faster response times.
4. **Simpler API Versioning**: Putting versioning into the resource path, like `/v1/users`, can smooth out the process of making changes without breaking existing endpoints. Juggling versioning with query parameters can get tricky on both the backend and for clients who use the API.
5. **Reducing the Chance of Duplicated Requests**: A well-organized, resource-based approach reduces the chance of accidentally creating the same request multiple times, which can happen with too many parameters. Every unique resource path is usually tied to a specific representation of a resource, making state management easier.
6. **More Meaningful Error Handling**: With clearer, resource-based endpoints, error responses can be designed more effectively. If a requested resource doesn't exist, a well-structured path can give more informative feedback compared to a convoluted query string.
7. **Cleaner API Documentation**: APIs that use resource-based URLs usually need less documentation compared to ones with many parameters, because the resource structure often tells you what's intended. This can make onboarding easier for new developers who interact with the API.
8. **Flexibility in Filtering and Sorting:** While resource paths make it easy to access core resources, combining them with well-defined methods for filtering and sorting offers the same level of control as parameters without cluttering the URL structure.
9. **More Consistent Client Behavior**: Resource-based paths can promote more consistent behavior across various API clients, lessening the risk of inconsistencies that can arise when clients interpret complex query parameters differently.
10. **Potential Security Advantages**: Simplifying URLs by focusing on resource paths instead of complex query parameters can make it harder for bad actors to see sensitive data, and reduce possible attack vectors like injection attacks since fewer parameters are exposed during communication.
Understanding Multiple Query Parameters in URL Design A 2024 Guide to RESTful API Best Practices - Multiple Parameter Sorting And Filtering Implementation Strategies
When designing REST APIs, a key aspect is efficiently implementing multiple sorting and filtering options for users. This is commonly achieved through query parameters – those extra bits of information attached to a URL. These parameters, formatted as key-value pairs, let clients specify complex filtering and sorting requests, making data retrieval much more flexible.
However, providing this flexibility requires careful consideration. Poorly designed parameter handling can lead to security risks or overly complex URLs that are difficult for both humans and machines to understand. It's important to consider the use of array parameters, for example, which provide more complex filtering capabilities, but come with a need to balance increased functionality against potential issues with URL length.
Ultimately, the goal is to strike a balance. We want to design APIs that are robust and provide the desired functionality, but that also retain clarity and simplicity. This ensures that the API remains user-friendly and easily maintained while still allowing clients to retrieve data in a way that suits their specific needs. There's a need to be mindful that the elegance of your design can be undone by poor implementation choices.
1. **Keeping an Eye on Performance**: How you implement sorting and filtering with multiple parameters can have a big impact on your API's speed. Well-designed queries can handle a ton of data, but poorly structured ones can bog down under pressure if the database isn't set up efficiently.
2. **Caching Gotchas**: If users can combine parameters in lots of ways, your cache can quickly get overloaded. It can become tricky to manage the growing number of cached responses, leading to inefficiencies if not considered upfront.
3. **How It Impacts Your Database**: The choices you make for sorting and filtering often have implications for how your database is structured. If the database isn't set up with good indexes for the columns being used in filters, it can significantly slow down your API.
4. **Making It Easy For Users**: URLs with too many parameters aren't very user-friendly. From a usability perspective, it's generally considered good practice to ensure users get a response within a fraction of a second – poorly designed parameters can easily make an API feel slow and unresponsive.
5. **Security Concerns**: When you're dealing with user-provided inputs for sorting and filtering, there's a higher risk of security problems like SQL injection. You absolutely have to make sure you sanitize and validate the inputs carefully to protect your API from attacks.
6. **Data Consistency Headaches**: Handling many parameters can make it trickier to guarantee your data is always consistent. If users can sort and filter across multiple, connected datasets, it's a lot harder to ensure transactions are handled properly without issues.
7. **Keeping Track of History**: If your API lets users sort and filter data, managing past versions of data can become complex. Different combinations of parameters can give users very different views of how the data looked at various points in time, adding a layer of complexity to data management and replication.
8. **Rate Limiting Complications**: Complex queries can easily lead to a big increase in API calls, which can create problems for rate limiting policies. You might need to rethink how you implement rate limiting to effectively protect your backend during peak usage.
9. **Client-Side Balancing Act**: When you have a lot of parameters, you need to consider how you manage client-side load balancing. If different combinations of parameters lead to an uneven distribution of requests across servers, it can cause issues with availability and response times.
10. **Thinking About Meaning**: The choices you make for your sorting and filtering parameters should make sense in the context of what your API is for. Users are more likely to embrace your API if the parameters they interact with reflect how they naturally think about the underlying data.
Understanding Multiple Query Parameters in URL Design A 2024 Guide to RESTful API Best Practices - Memory Optimization For High Volume Parameter Processing
When dealing with a large number of parameters in REST APIs, especially when queries involve substantial data processing, memory optimization becomes critical for maintaining good performance. Understanding how queries are executed, especially within database systems like those found in cloud environments, can guide efforts towards better optimization. Analyzing execution plans can be helpful for identifying and fixing bottlenecks.
Designing parameters in a clear and concise way is essential for both memory efficiency and user experience. APIs that are easy to read and understand are easier to optimize. Employing well-structured parameter calls, and using array-based structures for more complex filtering when appropriate, can help keep URLs from becoming overly cluttered. This can be particularly useful for clients processing the responses from the API.
The goal is to create APIs that are responsive and handle a large volume of data without consuming excessive memory resources. By implementing thoughtful strategies for parameter handling, developers can build APIs that are not only efficient but also pleasant for users to interact with.
1. **Keeping Memory in Check with Complex Queries:** Handling a large number of query parameters effectively can make a huge difference in how much memory your API uses. Using clever data structures like tries or hash maps designed for efficient parameter lookups can speed up processing while using less memory. This becomes more critical when scaling APIs to handle a huge volume of requests.
2. **Garbage Collection and Parameter Overload:** In languages with automatic garbage collection, like Java or C#, constantly creating and destroying objects to manage many query parameters can lead to more frequent garbage collection cycles. This can cause noticeable delays in API response times, impacting user experience.
3. **Bloated Memory and Unused Data:** APIs dealing with a lot of parameters can end up with "memory bloat"—where data that's not being used sticks around in memory, slowing everything down. Finding and fixing these inefficiencies can give a substantial boost to API responsiveness.
4. **Caching Parameters for Memory Efficiency:** Caching strategies specifically for query parameters can be incredibly valuable for managing memory usage. By storing the results of frequently used parameter combinations, APIs can reduce the need to repeatedly calculate the same thing, leading to faster response times.
5. **Memory Usage with Nested Structures:** When you have query parameters that involve nested structures or arrays, it can significantly increase memory usage. Before processing, simplifying or "flattening" these structures can help control the memory footprint, especially during periods of heavy usage.
6. **Connection Pooling and Memory Savings:** Many high-volume APIs use connection pooling to optimize memory management. Instead of establishing a new database connection for every query, these pools reuse connections, saving resources and improving API response times.
7. **Loading Data On Demand:** Lazy loading is a technique that can optimize memory usage by loading data only when it's needed, rather than loading everything at once. This is a helpful approach when managing resources during peak API activity.
8. **Investigating Memory Usage:** Regularly analyzing memory usage patterns can uncover performance bottlenecks related to parameter handling. Tools that monitor memory allocations can give insights into inefficient data structures or algorithms, allowing for targeted optimization efforts.
9. **Parameter Validation's Memory Cost:** Extensive parameter validation can consume more memory because each step may involve allocating additional memory. Streamlining the validation process and eliminating redundant checks can help mitigate this memory usage.
10. **Keeping an Eye on Memory Usage:** API systems often rely on monitoring tools to track how query processing impacts memory. This constant monitoring can signal when memory limits are approaching, letting you scale or optimize the system before performance issues arise.
Understanding Multiple Query Parameters in URL Design A 2024 Guide to RESTful API Best Practices - Security Best Practices For Query Parameter Validation
When building RESTful APIs in today's environment, properly validating query parameters is a key aspect of security. Failing to do so leaves your API open to attacks like SQL injection or cross-site scripting (XSS), where malicious code can be slipped into inputs and used to manipulate the API.
It's important to build safeguards into your design so you minimize the risk of these kinds of attacks. This includes preventing excessively long or complex query parameters, which can make it more difficult to understand what's happening and can create vulnerabilities.
Beyond that, having well-defined parameter names makes the API's intentions clear. This isn't just about making it easy to understand, it's also a factor in a more secure API design.
It's also essential to regularly review the security of how you deal with query parameters. Threats and attack techniques are always evolving, so a static security posture is not enough. By making security a part of the ongoing API maintenance process, you can adapt to changes and potentially mitigate the impact of any newly discovered vulnerabilities.
1. **The Sneaky World of Injection Attacks:** It's easy to think of just SQL injection when it comes to query parameters, but that's a narrow view. APIs are vulnerable to other injection techniques, too, like command injection, LDAP injection, and even XML injection, all of which exploit poorly validated input.
2. **Parameter Order: A Security Angle?** While the order of query parameters usually doesn't matter for the API itself, it can create hidden security risks. An attacker might try to change the order of parameters to exploit vulnerabilities if the validation logic doesn't properly check parameter positions and types in sequence.
3. **Data Type Mismatches: A Security Risk:** Many APIs don't enforce strict data type checking on parameters. Allowing unexpected data types—like getting a string when you're expecting an integer—can lead to crashes and also provide pathways for different attacks.
4. **Regular Expressions: Double-Edged Sword?** Regular expressions are a common way to validate query parameters, but poorly written regex can cause performance issues and introduce vulnerabilities. A pattern that's too broad can let malicious inputs through the validation process unnoticed.
5. **User-Agent Header: A Hidden Threat?** It's easy to overlook the User-Agent header, but this header can impact how query parameters are handled. Attackers could try to change the User-Agent header to make the backend system process query parameters in a harmful way based on the user's profile or implied device type.
6. **Mapping Parameter Journeys:** It's really helpful to have a good understanding of how query parameters move through your application. Tools that visualize the journey of parameters from the initial API request to the way they interact with databases can be really useful for finding weak points in how the data is processed.
7. **Using External Security Libraries:** Not every programming language has built-in features for parameter sanitization. Using dedicated security libraries can make query parameter validation more robust, but it's important to remember that those libraries themselves can sometimes have flaws that need to be patched.
8. **Query Parameters: A DoS Vector?:** Uncontrolled query parameters are a potential path for Denial of Service (DoS) attacks. An attacker can send lots of complex requests that overwhelm your API resources, preventing legitimate requests from getting processed.
9. **Rate Limiting and Parameter Security:** Good validation often works best with rate limiting. Strong rate limiting features can help stop abusive patterns that might exploit query parameters by sending a huge number of requests, especially ones that involve variations of otherwise harmless inputs.
10. **The Missing Pieces in Security Training:** While API security is getting more attention, there are still gaps in how developers are trained in secure coding practices related to query parameters. This lack of training can lead to mistakes in implementing critical validation techniques.
Understanding Multiple Query Parameters in URL Design A 2024 Guide to RESTful API Best Practices - Parameter Based URL Caching And Performance Tuning
When dealing with multiple query parameters in your REST API design, understanding how caching and performance tuning interact becomes crucial. Caching is a powerful way to improve the speed of your API, especially when certain combinations of parameters are used frequently. However, if you're not careful, having too many possible parameter combinations can create a massive cache that's difficult to manage and can even slow things down.
Building a good caching strategy for parameter-based URLs means finding a balance. You want to be able to cache frequently accessed data to speed things up for users, but you also don't want to create a bloated cache that's a resource drain.
In addition to caching, tuning the performance of your API when it comes to query parameters involves making smart decisions about how parameters are processed. This can affect how much memory your API uses, how long it takes to respond to requests, and the general efficiency of the entire process. The aim is to keep your API fast and responsive without sacrificing the ability for clients to craft varied requests through multiple parameters.
Ideally, you want a system where caching enhances the performance of the API without introducing new complexities or difficulties for those interacting with it. It's all about making your API as efficient as possible without sacrificing its flexibility.
1. **Caching Challenges with Parameters**: When URLs have lots of parameters, it can make caching more difficult. If similar requests differ only by a few parameters, the cache might not recognize them as the same, leading to lots of redundant cache entries. This can happen because many caching systems rely on the whole URL to decide if a request is a match.
2. **Impact on Load Balancers**: Using parameters can also complicate how load balancers distribute traffic across servers. If very similar requests differ only in their parameters, it becomes tougher for the balancer to spread out the workload evenly. This can lead to some servers being overloaded while others sit idle, creating uneven response times.
3. **Encoding Overhead**: Encoding URLs correctly for all those parameters can be more demanding than it looks. Each encoded character adds to the URL length, and that adds up quickly in APIs that see lots of traffic. This can impact both memory usage and processing time on the server.
4. **Memory Allocation Variations**: The way APIs handle parsing query parameters can impact memory allocation. If the patterns of the parameters aren't consistent, the server might end up allocating memory inefficiently.
5. **Documentation Complications**: Tools that generate API documentation may not be as helpful when there are lots of parameters. If parameters are deeply nested or vary a lot, the docs can become very hard to read. This makes it tougher for developers who are using the API.
6. **Logging and Overhead**: When every variation of an API call with different parameters is logged, it can generate a lot of data. This increases the storage needed for logs and makes it tougher to analyze them effectively. It also risks hiding important information needed to optimize API performance.
7. **Parameter Length Limits**: Some databases have limitations on the length of strings, which includes URLs with parameters. If you hit these limits unexpectedly, it might lead to query truncation, resulting in incomplete data being retrieved or API errors. It's essential to have checks for this.
8. **CORS Policy Interactions**: Cross-Origin Resource Sharing (CORS) is also impacted by how URLs are structured with parameters. If parameters are poorly constructed, they could introduce security issues if they change where a request is considered valid from.
9. **Proxy & Cache Interactions**: If your API uses proxies or caches, they might not behave the same way with URLs that have many parameters. This can cause issues with performance and cache hit rates. Understanding how these systems work is essential for maximizing response times.
10. **API Security Considerations**: The complexity introduced by numerous query parameters can increase the attack surface of your API. This creates more potential ways for attackers to exploit vulnerabilities. It also makes it harder to effectively monitor and limit the rate of requests to protect the backend, so security approaches need to be more sophisticated in these situations.
More Posts from :