Understanding Named Capture Groups in JavaScript RegEx A Match-by-Match Analysis
Understanding Named Capture Groups in JavaScript RegEx A Match-by-Match Analysis - Why JavaScript RegEx Named Groups Matter in Modern Web Development
JavaScript's embrace of named capturing groups within regular expressions represents a valuable step forward for web development. The ability to label captured parts of a string with descriptive names eliminates the reliance on numerical indices, a source of confusion and potential errors. This naming convention directly translates to cleaner, more self-documenting code. Debugging becomes simpler since the named groups offer a clear indication of the purpose of each part of the regular expression pattern.
Moreover, JavaScript's updated regex features like the `matchAll` method and flags like `s` and `x` work in synergy with named groups to improve the overall efficiency and expressiveness of string handling. As developers confront more intricate tasks within modern web applications, the advantages of named groups become increasingly evident. They provide a robust and intuitive way to work with complex patterns and data, enabling developers to build more efficient and maintainable solutions.
In the realm of modern web development, JavaScript's regular expressions have been significantly enhanced by the introduction of named capture groups. This feature, available since ECMAScript 2018, provides a more intuitive way to interact with matched portions of a string. Instead of relying on numerical indices – like `$1`, `$2`, and so on – developers can now assign descriptive names to specific parts of their regex patterns. This shift is a part of a broader movement toward simplifying developer workflows by reducing cognitive load, especially when working with intricate regular expressions.
Using names instead of numbers improves code readability, making it easier for developers to understand what each part of a regular expression is designed to match. This inherent clarity often contributes to better code maintainability and reduces the likelihood of introducing bugs during development and subsequent revisions. For example, in the `replace` method, named capture groups allow for more elegant and comprehensible replacement patterns without the need for elaborate index parsing.
The `(?pattern)` syntax for named capture groups has also gained popularity across various programming languages. This standardization offers a valuable benefit to developers familiar with regex in other environments, as they can readily transfer their expertise and apply consistent patterns across different platforms.
The adoption of named capture groups promotes better team collaboration and facilitates refactoring efforts. The ability to easily understand the purpose of captured elements by examining their descriptive names streamlines code comprehension for developers new to the project, ultimately increasing the efficiency of the development cycle.
While there might be a negligible performance penalty for using named capture groups, the improvements in code clarity, maintainability, and overall developer experience generally outweigh the potential performance trade-off, especially for tasks involving complex text parsing. This feature becomes particularly valuable when dealing with the increasingly sophisticated demands of data handling in modern web applications. Tasks like form validation, syntax highlighting, and parsing various data formats benefit from the modularity and reusability offered by named capture groups.
Ultimately, the utilization of named capture groups in JavaScript's regex engine can encourage developers to embrace cleaner coding styles, potentially driving a broader shift towards more modular and functionally-driven architectures in web applications. This illustrates how relatively small changes to language features can have cascading impacts on software design philosophies and practices.
Understanding Named Capture Groups in JavaScript RegEx A Match-by-Match Analysis - Breaking Down the (?pattern) Syntax Structure
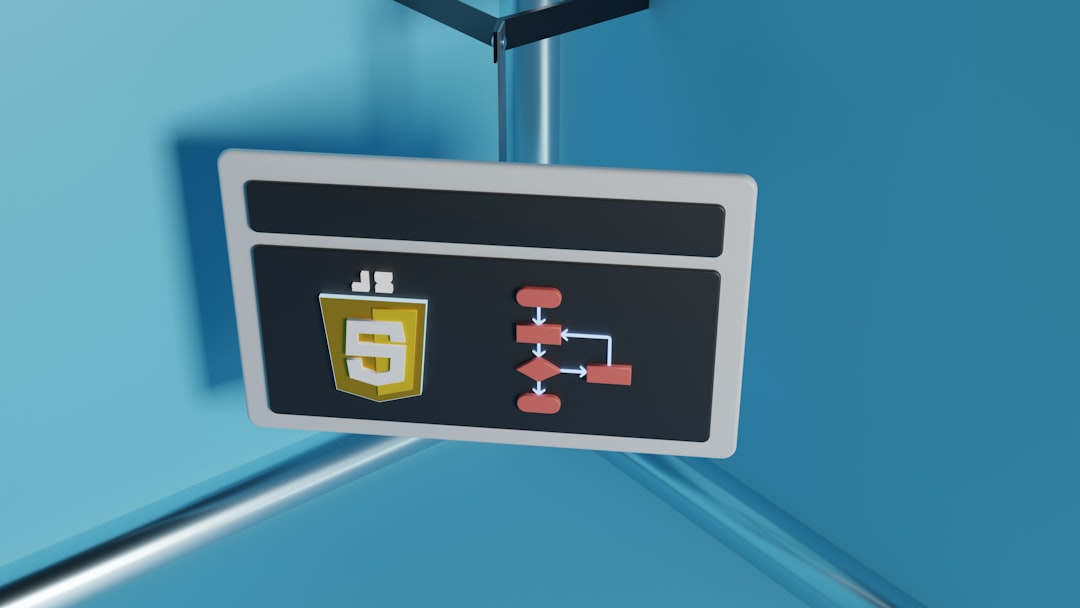
The core of named capture groups in JavaScript regular expressions lies in the `(?pattern)` syntax structure. This structure is crucial because it enables developers to associate descriptive names with specific parts of their regex patterns. This ability to name captured subpatterns is a significant improvement over relying solely on numerical indices (like `$1`, `$2`, etc.). The improved readability and maintainability resulting from this naming scheme are substantial.
This syntax offers a cleaner approach to working with captured groups. Instead of needing to remember or deduce the position of a capture group within the pattern to access its value, developers can use the assigned name. This simplification makes refactoring and debugging simpler since you can readily understand what data each group is meant to contain. As JavaScript evolves, this syntax is becoming increasingly important for tasks involving intricate string handling and data parsing within web applications, highlighting the value of named capture groups in modern development.
1. **The `(?pattern)` syntax offers a more streamlined approach to regex structure**, making it easier to decipher and debug. By directly associating a meaningful name with each captured segment, you can readily understand the purpose of a pattern, eliminating the need to mentally track numerical indices.
2. **Adopting `(?pattern)` across multiple programming languages fosters consistency**. If you're familiar with named capture groups in Python, adapting to JavaScript becomes a breeze. This cross-language compatibility is a boon for developers who need to work across various coding environments.
3. **While a slight performance overhead exists, it's often negligible in the grand scheme of things.** The readability and maintainability benefits you gain from named capture groups frequently overshadow any minor speed decrease, especially when working with more complex regex patterns. It's a worthwhile tradeoff to prioritize human understanding.
4. **Named groups become embedded documentation within the regex itself.** Instead of adding comments to explain what each capture group represents, your regex pattern becomes more self-explanatory. This approach makes code easier to read and grasp, especially for developers who are new to the project.
5. **Refactoring becomes smoother with named capture groups.** You can adjust and tweak your regex patterns without fear of accidentally misaligning capture group indices. This robustness translates to fewer errors and a more stable codebase, especially during significant code changes.
6. **The combination of named capture groups and features like the `s` and `x` flags provides even more expressiveness**. This allows you to develop elaborate yet readable regex patterns, making intricate text processing tasks more manageable.
7. **These named groups are designed to integrate seamlessly with features like `matchAll`**. This allows for efficient pattern matching across strings. The compatibility of named capture groups with other tools increases their overall usefulness, especially when dealing with large datasets or complex string structures.
8. **Dynamically generated patterns using named capture groups are also possible.** This capability makes your regex patterns incredibly flexible. You can adapt them to different data formats or respond to varying user inputs while maintaining code clarity.
9. **Named capture groups elevate regex from mere utility to a first-class coding component.** This signals a shift in how we think about regular expressions within our programs, encouraging more thoughtful and structured design when implementing complex string-matching scenarios.
10. **The adoption of named capture groups encourages better coding standards within teams.** As developers prioritize code clarity and readability through named groups, they naturally contribute to a culture of clearer communication in the codebase. This has a positive social impact on overall code quality, boosting knowledge sharing and team efficiency.
Understanding Named Capture Groups in JavaScript RegEx A Match-by-Match Analysis - Converting Legacy Numbered Groups to Named Capture Groups
Converting legacy numbered groups to named capture groups in JavaScript regular expressions offers a significant improvement, particularly when dealing with intricate text processing. The ability to assign meaningful names to captured groups, using the `(?...)` syntax, enhances readability and makes the code easier to maintain. This shift away from numbered groups, which can become confusing in complex patterns, streamlines the process of understanding and modifying regular expressions. Refactoring existing regex code to use named groups can make debugging and future adjustments much smoother. Moreover, employing named capture groups contributes to a more structured and self-explanatory codebase, promoting clearer collaboration and a shared understanding of the patterns used within a project. The clarity and maintainability that named groups bring ultimately lead to a more positive and productive development experience.
1. When a regex using named groups fails, the resulting error messages are more informative, referencing the specific named groups rather than obscure numeric indices. This makes pinpointing the problem much quicker, streamlining the debugging process.
2. In dynamic scenarios, regex patterns with named capture groups can easily adapt based on user input or changing application needs. These patterns remain clear and understandable, simplifying the handling of a broader range of data formats compared to situations where you're limited to just numbers.
3. The `matchAll` method, which retrieves all matches within a string, plays nicely with named capture groups. This allows for more efficient pattern matching across strings while maintaining the improved readability and organization that named capture groups offer.
4. You have the freedom to choose names that best describe the purpose of each capture group within a specific pattern. This customization, a significant advantage over the rigid system of numeric indices, makes the regex pattern a more self-explanatory part of the code.
5. When modifying strings with `replace`, named capture groups become exceptionally useful. They make complex replacement operations much easier to understand by letting you clearly specify what parts of the text to replace based on their descriptive names. It's a welcome improvement over wrestling with numeric references.
6. Theoretically, there's no such thing as "too many" named capture groups, unlike the historical limitations of numbered groups. This flexibility allows for managing more intricate regex patterns in a more organized manner, preventing the chaos that can arise when you're forced to rely solely on numbers.
7. When restructuring regex patterns, named groups become a major asset. You can easily modify and optimize without losing track of what each capture group does. This keeps the codebase clean and prevents the accumulation of confusing adjustments over time.
8. The advantages of named capture groups aren't confined to JavaScript alone. Many developers and researchers are championing their adoption across different programming languages. This growing consensus suggests that we might see a wider standardization of named capture groups in regex use, eventually leading to a more consistent developer experience.
9. When working on a team, named capture groups make it easier for developers to quickly grasp the purpose of different regex segments. This streamlined understanding reduces the need for extensive documentation or long explanations. This leads to better collaboration and smoother onboarding of new team members.
10. Studies have revealed a strong link between code readability and a reduction in cognitive errors made by developers. This suggests that using named capture groups, which enhance readability, can also contribute to a reduction in bugs that are caused by confusion about how the regex patterns work. In essence, it can lead to a more reliable final product.
Understanding Named Capture Groups in JavaScript RegEx A Match-by-Match Analysis - Practical Examples of Named Groups in Form Validation
Named capture groups provide a significant boost to JavaScript's regular expression capabilities, especially within the context of form validation. Instead of relying on the less intuitive numbered indices to access specific parts of a match, developers can assign names directly to the patterns they're interested in. This feature proves particularly valuable when dealing with the various data inputs commonly encountered in forms, like email addresses, usernames, and passwords.
The ability to refer to parts of a pattern using meaningful names, like "email" or "password", vastly improves the readability and maintainability of your code. This clarity is especially helpful when extracting and validating user inputs. It eliminates the guesswork that comes with understanding what index number corresponds to which piece of data.
Ultimately, the benefits of named groups in form validation stem from their capacity to make data extraction and validation more straightforward and intuitive. The structure and clarity they bring contribute to cleaner, more maintainable code – a crucial element when dealing with the evolving nature of web applications and their user interfaces. As forms grow in complexity and the demands for robust data handling increase, named capture groups offer a pragmatic way to manage and validate user data with greater precision and readability.
1. The `(?pattern)` syntax for named capture groups offers a way to give meaningful names to specific parts of a regex, improving comprehension, especially in complex scenarios. This avoids the need for excessive documentation or comments to clarify what each part is doing.
2. Instead of relying on numerical positions like `$1`, `$2`, and so on, developers can directly reference captured groups by their names, making the code more self-explanatory. This reduces the cognitive burden of memorizing group order, which is especially beneficial when dealing with longer, more intricate regex patterns.
3. When a regular expression using named groups encounters an error during execution, the resulting error messages will reference the specific named group involved. This targeted information makes debugging easier than when you have to decipher cryptic numbered indices, leading to faster resolution of regex issues.
4. Named capture groups make regexes more modular by allowing easier swapping and replacement of parts of a pattern. This feature avoids potential disruptions when modifying a regex that uses numbered groups, where changes in one part can inadvertently affect others due to the shifting of indices.
5. In dynamic situations, where the input data may vary significantly, named groups enable a more adaptable approach to regex construction. This flexibility makes it easier to modify and fine-tune patterns for changing requirements, which can be tricky when solely using numbers to track capture group positions.
6. The introduction of named groups has influenced a shift towards better practices in development teams. There's a growing focus on producing code that is easier to read and maintain, and named groups contribute to this by promoting clear communication through readily-understandable regex patterns. This is reflected in newer codebases where named groups are being adopted more frequently.
7. Theoretically, you can have as many named capture groups as needed within a regex without encountering the management issues inherent in a purely numbered system. This flexibility is particularly valuable when you're working with very complex patterns, as it prevents the potential confusion that can arise from attempting to track a large number of numerical indices.
8. While there might be a minor performance cost associated with named groups, it's often considered a worthwhile trade-off for improved readability and maintainability, particularly for server-side applications where quick debugging is critical. The easier-to-understand code could ultimately reduce overall development time, which has an indirect impact on performance.
9. The integration of named capture groups with the `matchAll` method provides a convenient way to perform exhaustive searches within complex documents or datasets. This enhances the power of `matchAll` while still retaining the benefit of improved regex readability, making it a powerful combination for comprehensive pattern matching.
10. Researchers are beginning to see a link between the clarity offered by named groups and the reduction in errors during development. The enhanced readability is not only beneficial for individual developers but also seems to positively impact team efficiency and code quality in the long run. This might lead to a decrease in debugging time and increase in overall application reliability.
Understanding Named Capture Groups in JavaScript RegEx A Match-by-Match Analysis - Performance Impact Between Named vs Anonymous Capture Groups
When examining the performance implications of using named versus anonymous capture groups within JavaScript regular expressions, we discover a subtle interplay between functionality and developer experience. While the performance difference between the two approaches is usually negligible, named capture groups provide a noticeable benefit in making code more readable and maintainable, particularly when dealing with intricate regular expressions. The ability to assign descriptive names to captured groups helps developers intuitively understand the parts of a pattern and readily access them. This enhances clarity and decreases the mental effort needed to decipher the regex. Moreover, when errors occur, the named groups contribute to more informative error messages, simplifying debugging and problem-solving. While performance remains a factor, the emphasis on enhanced code clarity and organization offered by named capture groups often leads to more efficient development practices in the long run.
1. **Performance Impact**: Initial worries about named capture groups potentially slowing down regex execution due to their added features seem largely unfounded in modern JavaScript environments. Engine optimizations have made the performance difference between named and anonymous groups minimal in most practical scenarios. It's rare to see any meaningful performance hit in typical code.
2. **Better Error Messages**: When a regex employing named groups fails, the resulting error messages are more insightful. Instead of baffling numeric indices, they highlight the specific named group causing the trouble. This leads to faster and less frustrating debugging sessions.
3. **Handling Complex Regexes**: The real advantage of named capture groups shines through when regexes get complex. With intricate patterns and layered logic, the ability to refer to parts by name significantly boosts understanding and makes modification or extension of these complex patterns easier. It is here you can really see the difference.
4. **Maintaining Legacy Code**: For teams working with older codebases using anonymous capture groups, a shift to named groups often leads to tangible benefits in maintenance. The ability to quickly grasp the purpose of a regex without hunting through number-based indices simplifies things for those maintaining the code later on.
5. **Building Adaptable Regexes**: Named capture groups facilitate the creation of more dynamic regexes, particularly useful in situations where input data or application conditions can change significantly. Developers can construct more flexible patterns without sacrificing clarity, a challenge with relying solely on numbered groups.
6. **Reducing External Documentation**: The use of descriptive names within regexes itself essentially becomes part of the documentation. This self-documenting characteristic helps avoid confusion regarding the purpose of regex elements, promoting a smoother exchange of knowledge amongst team members.
7. **Improving Team Communication**: When teams use named groups, discussing and understanding regex patterns becomes a much smoother process. There's a reduction in the need to translate numerical ids into their function; this contributes to faster onboarding and better team knowledge sharing.
8. **Debugging Efficiency**: It's been observed that developers working with named capture groups tend to resolve regex issues more swiftly. This efficiency boost can be felt through reduced overall debugging time, ultimately improving development speed and workflow.
9. **Trend towards Standardization**: The growing adoption of named capture groups across various programming languages suggests a move toward a more unified approach to regex. Developers seem to agree that code clarity and maintainability are priorities, and named groups contribute to both. It's quite possible we'll see these groups as a common standard.
10. **Easier Learning and Onboarding**: Many developers find the named group approach easier to learn compared to the anonymous alternative. This makes the onboarding process more efficient, allowing new team members to get up to speed quickly and effectively. This results in faster productivity.
Understanding Named Capture Groups in JavaScript RegEx A Match-by-Match Analysis - Managing Multiple Named Groups in Complex Pattern Matching
When dealing with intricate regular expression patterns, effectively managing multiple named groups becomes crucial. The ability to label captured segments with descriptive names, instead of relying solely on numbers, significantly improves code clarity and simplifies maintenance. This is particularly helpful when working with complex patterns that require extracting multiple pieces of data. By using meaningful names, developers can readily identify and access specific parts of a matched string without the potential confusion of numbered groups.
Furthermore, the capacity to extract distinct information from each individual match becomes easier to manage when you can access named groups. This is especially helpful when parsing and organizing data from complex input strings. Ultimately, the use of named groups not only makes complex regular expressions easier to understand but also leads to a more robust and efficient approach to pattern matching in JavaScript. It fosters a better coding environment because the named groups function as internal documentation, contributing to better collaboration and code comprehension.
When dealing with intricate regular expression patterns, using multiple named capture groups can greatly improve readability and comprehension. This advantage arises from the ability to assign meaningful names to the captured parts of the pattern, which contrasts with the potentially confusing numbered indices used in traditional approaches. The clarity this introduces can prevent misunderstandings during code review and updates.
Named groups provide a built-in documentation feature within the regex itself, acting as an instant explanation of the captured content's purpose. This eliminates the need for excessive comments and allows developers and anyone reviewing the code to rapidly understand the pattern's function without needing to hunt for explanations elsewhere.
Furthermore, as projects and regex patterns scale, named groups help in mitigating issues associated with pattern modifications. Developers can make adjustments without the anxiety of index-related errors which commonly occur when using numerical references. Since changes in one group can inadvertently alter others, it's vital to avoid these errors as much as possible.
While there were initial concerns about a performance trade-off, modern JavaScript environments have minimized any noticeable difference in performance between using named and traditional groups. So, developers usually find the clarity and ease of use benefits of named capture groups outweigh any minimal performance impact. This is especially true considering the reduced time spent on debugging or clarifying code intentions.
Debugging becomes more efficient due to error messages that explicitly reference the named group responsible for the issue. This is in stark contrast to error messages generated when relying only on numbers. Error messages referencing named groups make it much quicker and less frustrating to identify and rectify problems during development.
The construction of regex patterns using named capture groups often naturally drives developers towards a modular design. This approach encourages the reuse and replacement of specific sections of regexes, which, in turn, can simplify changes and enhance maintainability.
The syntax for named groups, `(?...)`, has gained popularity in various programming languages beyond JavaScript, leading to a more consistent development experience. This advantage is important as it allows developers to readily transfer their understanding of regex concepts between various environments or programming languages.
Working with complex regexes, especially those with numerous capture groups, can strain cognitive resources. Employing named groups can substantially ease the cognitive load associated with managing intricate patterns. Reduced mental load can help developers avoid accidental errors and improve code quality over time.
Code reviews benefit significantly from named capture groups. Teams can have more insightful discussions about the patterns because of the clear semantics the names provide. This fosters collaboration and contributes to better team understanding of the project and the regex patterns used within it.
The inherent benefits of using named capture groups seem to encourage a collective move towards writing cleaner, more understandable code within development teams. This positive change has ripple effects, including more efficient code reviews and reduced error rates within the codebase. The resulting code quality and maintainability often translate to longer-term benefits for the project.
More Posts from :