7 Proven Ways to Debug Your Code A Practical Guide for Beginner Programmers
7 Proven Ways to Debug Your Code A Practical Guide for Beginner Programmers - Print Statement Strategy Breaking Down Complex Functions Line by Line
When tackling intricate functions, the "Print Statement Strategy" proves quite helpful. It's like dissecting a complex machine, one part at a time. By inserting print statements at strategic locations within the function's code, you can essentially peek inside and observe what's happening at various stages. This allows you to better understand the function's internal logic and flow. You can witness the progression of data and potentially uncover the source of errors or unexpected behavior.
While beneficial, this approach isn't without its pitfalls. Too many print statements can create a messy codebase, making it harder to read and understand. Also, sifting through a plethora of printed output can become a challenge in itself, potentially hindering rather than assisting your debugging efforts. Therefore, it's crucial to employ this technique strategically and sparingly. Ideally, print statements should only be used in areas where you suspect problems, to avoid cluttering up the entire codebase. It's wise to pair this approach with other debugging strategies to ensure a more effective and clear-cut debugging experience.
Debugging with print statements is a fundamental approach that has endured across programming generations. Its simplicity and ability to dissect the flow of execution make it a valuable tool, especially when dealing with intricate functions.
By strategically placing print statements at crucial points within a function, like before and after loops or conditional blocks, we gain insights into the code's decision-making process. This 'divide-and-conquer' tactic effectively breaks down complex functionality into more manageable chunks. It’s often through this kind of meticulous dissection that we stumble upon the root of unexpected behavior.
However, it's not without its caveats. Overusing print statements, especially in resource-constrained environments, can lead to performance degradation. Furthermore, interpreting the output in environments with concurrent execution can be tricky, as interleaved print statements can obscure the true order of events.
Despite these limitations, it's fascinating how many experienced programmers continue to rely on print statements, especially for larger codebases. They find it quicker and more intuitive to trace variable values directly via print statements compared to using elaborate debugging tools. Furthermore, print statements can foster a deeper understanding of a code's behavior, which is crucial for learning and skill development.
This "print debugging style" isn't just a haphazard approach. Many developers cultivate a method of producing highly readable and organized print outputs, almost resembling logging practices. This practice aids significantly in problem identification, even in sprawling codebases, where finding the needle in a haystack is a common debugging challenge. The essence of effective print debugging lies in deliberate placement and intelligent output formatting. It's a testament to the fact that simple tools, when used strategically, can provide profound insights.
7 Proven Ways to Debug Your Code A Practical Guide for Beginner Programmers - Setting Strategic Breakpoints Using VS Code Debug Mode
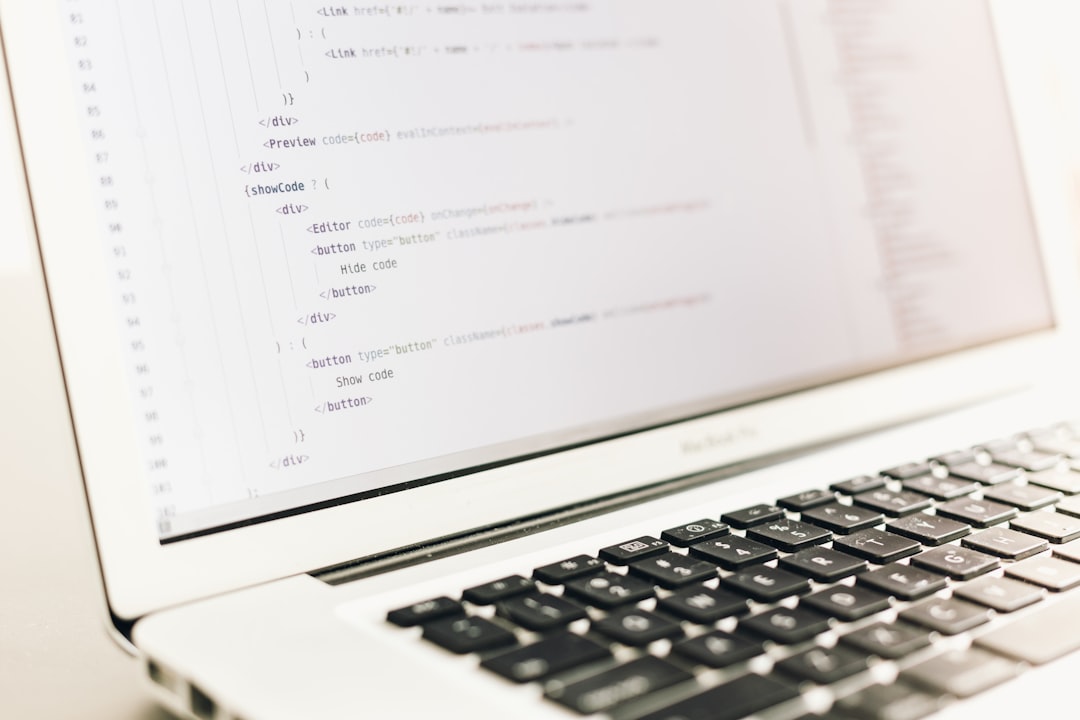
VS Code's debug mode, with its breakpoint functionality, lets you gain a deep understanding of your code's execution flow. By strategically placing breakpoints—essentially pausing execution at specific lines—you can closely examine the current state of your variables and how the program logic is unfolding. This hands-on control becomes even more effective when paired with a correctly configured `launch.json` file, which is vital for ensuring your breakpoints work properly, especially for applications built with frameworks like React or Next.js.
However, it's crucial to remember that breakpoints can only be set on lines containing executable instructions. This means you can't set them on simple declarations or signatures, potentially limiting their application in certain situations. The Debug Sidebar in VS Code serves as your control center for the debugging process, offering intuitive controls for starting, stopping, and pausing the execution. Mastering its functionalities can significantly improve your debugging efficiency and make the entire process less cumbersome.
VS Code's debug mode offers a powerful way to set breakpoints, effectively pausing code execution at specific points to gain insights into the program's state. While simple in concept, it's a cornerstone of effective debugging. You can essentially "freeze-frame" your program at precisely chosen moments, scrutinizing variable values and the flow of control.
The placement of breakpoints is crucial. Ideally, they should be strategically positioned in areas of suspected issues rather than indiscriminately scattered across the code. It's akin to setting traps in a complex system to catch the source of problems. This approach helps you sidestep painstakingly stepping through every line.
While basic breakpoints are a starting point, the debugger's capabilities extend beyond simple pauses. You can establish "conditional breakpoints"—breakpoints that only trigger when specific conditions are met. This comes in handy when bugs manifest only under specific circumstances.
Another interesting aspect is the "logpoint" feature. Instead of stopping execution, these logpoints print information to the console, effectively serving as a form of in-line logging. This can be useful in production environments for monitoring without introducing major performance bottlenecks.
In addition to these breakpoint features, the debugger lets you peer into the function call stack—a kind of historical record of function calls leading up to the breakpoint. This context is important for unraveling intricate interactions in a program.
The concept of variable watchers is another useful feature. During a debugging session, these watchers provide a live feed of variable values, essentially a window into how variables change during runtime. This is particularly insightful when tracking unexpected variable changes.
There's also support for multi-threaded debugging, crucial for understanding intricate multithreaded programs. The ability to set breakpoints in separate threads helps isolate the thread that's causing trouble.
Debugging is not just about pausing code—it's also about the ability to adapt and make changes on the fly. VS Code enables live code editing in some scenarios, allowing you to tinker and test modifications during a debugging session. This makes for a much more iterative development cycle.
The debugging features of VS Code extend even to the realm of test-driven development. Integrating with testing frameworks, you can step through both test code and application code concurrently. This tight integration fosters a very precise approach to debugging.
Furthermore, VS Code allows for customized debug configurations through the `launch.json` file. This means that you can tailor debug settings for various projects and needs. This configurability makes VS Code a versatile debugging environment.
In summary, setting breakpoints in VS Code provides an efficient way to track down those pesky issues that arise in coding. When used in combination with other techniques and features within the IDE, you can gain significant insights into program behavior and swiftly pinpoint and resolve bugs.
7 Proven Ways to Debug Your Code A Practical Guide for Beginner Programmers - Memory Leak Detection Through Chrome Developer Tools
Memory leaks, a silent performance drain in web apps, can be effectively hunted down using Chrome's Developer Tools. The Memory panel within DevTools is your starting point. It allows you to monitor memory usage over time, helping you identify potential leaks. Common culprits include JavaScript code that fails to release DOM elements it no longer needs, or situations where closures keep hold of variables that should be garbage collected.
To get a clearer picture of what's happening, the "3 Snapshot Technique" is useful. This involves taking multiple heap snapshots at different points in the app's lifecycle. By comparing these snapshots, you can see how memory is being allocated and identify leaks as areas where memory usage unexpectedly increases. Chrome's Performance Profiler provides a visual representation of memory consumption, making it easier to understand how memory use fluctuates during user interaction.
For digging deeper, the Memory Inspector is incredibly helpful. It allows you to delve into large data structures like arrays and view their contents, simplifying the process of identifying the source of memory problems. Testing is also valuable for debugging memory leaks. You can intentionally create scenarios that might lead to memory leaks, like repeatedly adding large amounts of data to an array, and observe how the memory panel reacts.
By mastering these tools within Chrome's DevTools, you can more effectively prevent and debug memory leaks, which are crucial to maintain a healthy and efficient web application. While memory leaks might seem complex to beginners, with consistent practice these debugging techniques become more intuitive and ultimately ensure your web application doesn't succumb to sluggishness due to unnecessary memory consumption.
When a web page steadily consumes more memory over time, it can lead to a situation called a memory leak. This can cause noticeable performance hiccups for the user, making the browsing experience less than ideal. Thankfully, the Chrome Developer Tools, or DevTools, have a specialized Memory panel tucked away within the action bar or the more expansive options menu.
This Memory panel is a developer's best friend when it comes to tracking memory usage over time. It helps pinpoint potential memory leaks in JavaScript applications. Often, memory leaks stem from scenarios where references to DOM elements aren't released when they're no longer needed or where closures unexpectedly cling to variables they should have let go of.
A handy technique in DevTools is the "3 Snapshot Technique". This method involves capturing a series of heap snapshots at strategic moments during the web page's lifecycle. By comparing these snapshots, you can analyze memory allocation and potentially track down leaks. This allows developers to literally see which parts of the code aren't releasing memory as they should.
DevTools also offers a Performance Profiler. This tool visualizes memory usage as a dynamic graph, which is particularly useful for tracking how memory consumption evolves over time during user actions. It helps to visualize memory consumption in real-time scenarios, offering a better sense of the actual impact of the application's code on the available system resources.
Then there's the Memory Inspector in DevTools. It can be a lifesaver when tackling large datasets. It simplifies the process of navigating through vast arrays and comprehending the contents of the memory, which in turn, assists in debugging those stubborn memory issues that may appear.
Ideally, to uncover memory leaks, we try to capture memory usage while performing specific application tasks. Adding and removing tasks or data entries can reveal unexpected increases in memory usage, hinting at possible leaks. If memory does grow unexpectedly when there's no real need to, it's often an indicator of a potential leak.
Recognizing typical memory leak patterns is crucial. Preventing these leaks is vital for building efficient and responsive web applications. Developers need to understand what leads to leaks and take preventive measures during the coding process.
To better understand memory leaks, it's sometimes helpful to induce them on purpose. You can simulate leaks for testing by setting up scenarios that cause memory consumption to grow uncontrollably. One approach could be repeatedly adding massive strings to an array. This lets you experiment and see how the browser's memory management system reacts in such contrived scenarios.
It's remarkable how the DevTools features enable us to delve into such aspects of program behavior that might seem out of reach initially. It's fascinating to see how the tools help visualize and understand those tricky scenarios that otherwise could be hard to grasp. While simple tools are sometimes overlooked, their ability to offer insights into seemingly complex issues is truly valuable and a critical tool in the debugging toolkit.
7 Proven Ways to Debug Your Code A Practical Guide for Beginner Programmers - Log File Analysis With Python Debug Module
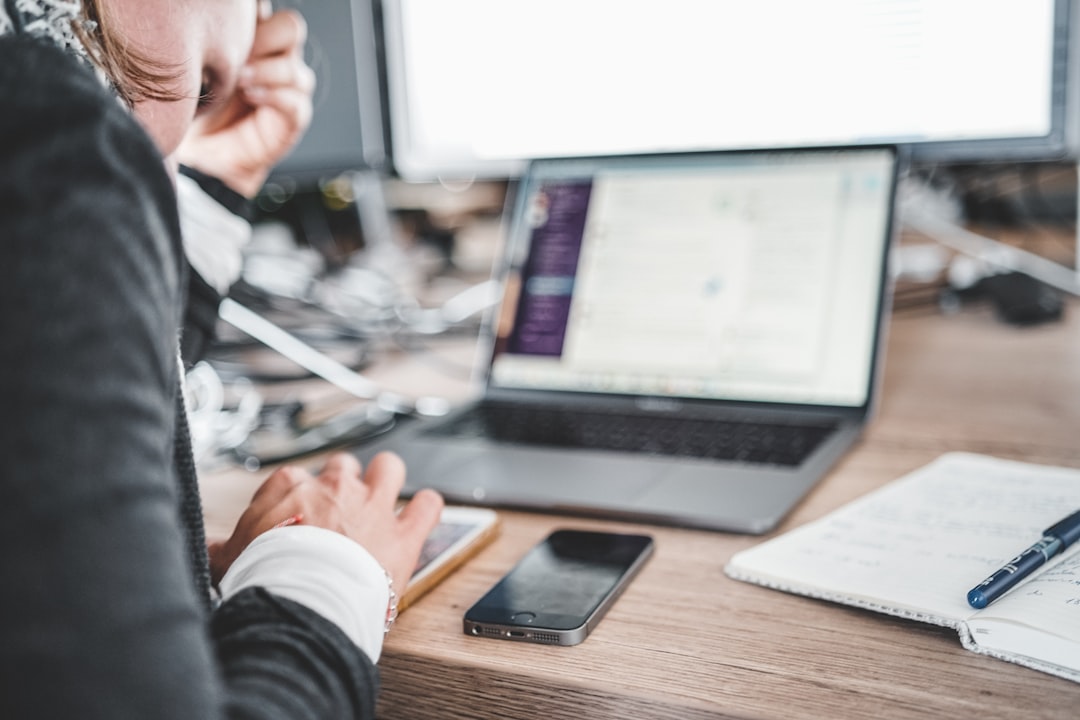
When dealing with complex software, understanding how your code behaves is crucial. Python offers the `logging` module as a valuable tool for capturing information about what your program is doing. This is especially helpful when things go wrong and you need to trace the problem. By setting different log levels, you can control the amount of information captured, ranging from detailed debugging messages to critical errors. Often, it's convenient to direct this output to a file, making it easier to review, particularly in larger projects where the console can quickly become overwhelmed.
However, logs themselves can become complex and difficult to parse if the issue isn't obvious. Here's where Python's Debug module, `pdb`, can help. `pdb` allows you to step through your code line by line, examine variables as they change, and get a more nuanced picture of what might be causing problems. This interactive approach, when paired with log file analysis, creates a powerful debugging strategy. It can significantly reduce the time spent troubleshooting by providing a deeper understanding of how the code works during runtime. While this might seem complex for beginners, learning how to analyze logs and effectively use the Python debugger is an investment that pays off in a significant way when building reliable software.
Python's `debug` module, often used for log file analysis, provides a unique way to interact with a program during its execution. It lets you peer into the program's state at any point, offering insights that you can't get from simply analyzing the code itself. This interactive approach can unearth hidden details about how the code behaves.
One of the module's strengths is the `trace` function, which allows for detailed, line-by-line tracing of program execution. Not only does this show you the flow of the code, it also reveals the frequency of function calls, which can be handy in finding performance bottlenecks.
A less obvious benefit is that log file analysis with `debug` is great for real-time debugging. You get immediate feedback about your program as it runs, unlike traditional logging, which only collects information after execution.
While most associate debugging with fixing errors, the `debug` module can actually deepen your understanding of your code's logic. This deeper comprehension of how your code works can shape how you write code in the future.
The `debug` module even allows you to load new code dynamically during runtime. This opens the door to quicker development cycles as you can test changes immediately, giving you a more agile workflow.
Interestingly, the `debug` module works well with other debugging approaches, like using loggers. This synergy between different debugging techniques allows for a more robust and efficient debugging workflow.
One surprising aspect of `debug` is its potential for identifying security vulnerabilities. By tracking data flow through the application, you can potentially spot security risks. This extends its usefulness beyond traditional debugging into the realm of code security.
Despite its capabilities, new programmers sometimes find `debug` complex and shy away from it. But once mastered, it can dramatically simplify the debugging process and improve your comprehension of data manipulation within your code.
In fact, many experienced programmers consider `debug` an integral part of their development process, much like version control. It's become a crucial tool for them in writing efficient code.
Finally, combining `debug` with logging allows for incredibly detailed reports on program execution, especially helpful in post-mortems when code malfunctions. This detailed information is invaluable when a team needs to troubleshoot problems and improve code quality systematically.
7 Proven Ways to Debug Your Code A Practical Guide for Beginner Programmers - Unit Testing Implementation With Jest Framework
Unit testing is a vital part of creating reliable code, and Jest makes it approachable for new programmers. Developed by Facebook, Jest's design emphasizes ease of use, which is a plus for beginners. Integrating it into your project is simple – you just install it using `npm` or `yarn` and add a basic test command to your `package.json` file. It handles JavaScript and TypeScript, making it versatile. It even has helpful features built-in like mocking and code coverage reports, which can be quite handy. It works well with frameworks like React, so it's a good choice for web development projects. In essence, Jest provides a gentle on-ramp to the world of unit testing. By learning how to use Jest, beginners can develop better coding habits and make debugging their programs less of a hassle. This ultimately leads to more robust code in the long run.
Jest, a testing framework crafted by Facebook, is designed with simplicity and ease of use in mind. It can be easily integrated into your project by installing it using either `npm install --save-dev jest` or `yarn add --dev jest`. You can streamline the testing process by adding a script to your `package.json` file like this: `"scripts": { "test": "jest" }`. Interestingly, it handles both JavaScript and TypeScript, making it versatile for many types of projects.
Furthermore, it comes equipped with some useful features, including built-in capabilities for mocking, coverage reports, and a wide range of comparison functions for assertions. It plays nicely with popular frameworks like React, Angular, and Vue, as well as projects using Babel. One of its most appealing traits is automatic mocking, which simplifies tests by automatically generating mock implementations for imported libraries, saving you from writing a lot of repetitive code.
A typical Jest test is set up with a file, such as `mathtest.js`, placed in a designated `tests` folder. Running the test suite is as simple as executing `npm test` from your terminal. It's particularly well-suited for unit testing in React applications, often leveraging a default `App.test.js` for testing components. It provides a straightforward interface, making it particularly friendly for beginning programmers to dive into the world of unit testing.
However, one of the less discussed aspects is Jest's inherent parallel nature which seems to boost test execution speed. Tests run in parallel by default, resulting in substantial performance improvements, especially when dealing with numerous tests. A common point of contention is "Snapshot Testing", where it essentially takes a picture of a UI's output and saves it to a file. This allows you to compare if any unintended UI changes have occurred.
Jest also shines when it comes to mocking and controlling dependencies during testing, simplifying test isolation. The built-in code coverage reports are useful for visually understanding which parts of the code are tested. One could argue that its "zero-config" approach is a key factor in its popularity for developers new to testing, streamlining initial configuration.
It seamlessly works with many common continuous integration tools which is a must in contemporary software development. While it's closely associated with React, it isn't limited to just React applications, it's also valuable for testing various JavaScript and TypeScript projects using frameworks such as Vue, Angular, and Node.js. Its healthy community provides resources, support, and timely updates. The clarity of the test structure and syntax helps developers easily write and maintain tests. Interestingly, it also simplifies asynchronous testing using `async`/`await` and promises, easing a common challenge.
In summary, Jest's combination of speed, ease of use, and comprehensive features makes it a valuable tool for unit testing, making it a worthy contender for novice and experienced developers alike. While there's still room for improvement, its focus on simplicity, readability, and extensibility makes it a practical and effective debugging method when used judiciously.
7 Proven Ways to Debug Your Code A Practical Guide for Beginner Programmers - Browser Console Methods For Frontend Debugging
The browser's built-in console, often accessed by pressing F12, is a valuable resource for debugging issues within the front-end code of a website or web application. It's a powerful tool because it allows developers to directly interact with their JavaScript code while the page is loaded in a browser. Fundamental methods like `console.log` are commonly used to quickly print information to the console during runtime, assisting in understanding the flow of data through the code. The `debugger` statement offers a more advanced way to pause execution, essentially acting like a breakpoint, which lets you inspect variable values and code execution flow at a particular point. Other console methods exist to output different types of messages, including error reporting using `console.error` or warnings using `console.warn`, to help guide the debugging process.
While these core methods are useful for many situations, some issues can be tricky to troubleshoot using just `console.log` statements. Advanced debugging techniques involve features like asynchronous debugging. These features become essential when dealing with code that runs in the background or at unpredictable times, making it hard to follow with just simple logging. For instance, dealing with `async`/`await` or Promises requires different strategies to track execution effectively.
In situations where the JavaScript code has been minified for production (e.g., to reduce file size), it's often impossible to understand the code without special tools. Source maps help to bridge this gap by translating the minified version back into its more readable original format, which is incredibly valuable for troubleshooting in real-world scenarios.
For any developer who works on the front-end, understanding the various console methods and mastering the ability to strategically use them is vital. It helps developers to approach debugging in a more efficient and systematic way, making the debugging process significantly less stressful and ultimately improving their ability to create higher-quality web applications.
The browser console, accessible through the F12 key, is a valuable tool for debugging frontend code. It allows you to view errors and warnings and even execute JavaScript commands directly, offering a quick way to inspect your application's state. `console.log` and the `debugger` statement are fundamental debugging tools, with `console.log` being useful for quick logging and `debugger` for pausing execution and examining variables in detail.
The `console` object in JavaScript comes with a set of helpful debugging methods, most of which are compatible with both web browsers and Node.js environments. However, it's important to realize that the methods available might vary between browsers and might not be completely cross-compatible. More advanced debugging approaches, like leveraging async methods, can be crucial when `console.log` isn't enough to pinpoint the cause of an issue in a complex execution path.
When dealing with minified JavaScript files from production builds, having source maps available is essential for effective debugging. The source maps essentially translate the minified code back to its original, human-readable state, helping developers track down the problem more efficiently. `console.debug` acts like `console.log` but is typically intended for more granular debugging information, which can sometimes get lost within the sheer volume of `console.log` output.
Using linters and code analyzers can greatly improve the debugging process. These tools flag potential problems early in the development phase, making debugging less painful in the later stages. While these tools can be immensely helpful, they are not a substitute for critical thinking and problem-solving skills. Debugging often requires teamwork and collaboration. It’s a good idea to ask for assistance from other developers if you hit a roadblock. It's often through a second set of eyes that a subtle problem becomes apparent.
Becoming proficient with the various `console` methods and knowing when to utilize each one effectively can greatly enhance the debugging experience for frontend developers. For example, sometimes `console.warn()` is a better option than `console.log()`, especially when you suspect a problem. Mastering the art of effective debugging is not just for senior developers. It's a vital skill for programmers at all levels, leading to a smoother and more efficient development workflow. There's a certain level of craftsmanship involved in debugging, and the tools themselves, like the console, are a fundamental part of a developer's craft. It's a skill that improves over time as your experience and intuition regarding how code behaves expands.
7 Proven Ways to Debug Your Code A Practical Guide for Beginner Programmers - Rubber Duck Method With Systematic Code Walkthrough
The Rubber Duck Method, a surprisingly effective debugging technique, involves explaining your code line by line to an inanimate object, often a rubber duck. The act of verbalizing the code's logic, purpose, and intended behavior forces you to think critically about each step. It's like having a conversation with a very patient, and completely uncritical, audience. This process helps to clarify your own understanding of the code, revealing hidden assumptions or inconsistencies that may be causing errors.
You essentially walk through your code, explaining what each section should do, what it's supposed to achieve, and the expected outcomes. The duck, being a non-judgemental listener, encourages a detailed and methodical explanation. You might find that, as you explain things aloud, you suddenly realize where you've made a mistake or overlooked a crucial detail.
While it might seem odd to talk to a rubber duck, the true power of this method lies in the act of verbalizing your thoughts and processes. It's surprisingly easy to miss logical errors when just reading through code silently. Forcing yourself to speak the code's logic out loud makes you engage with it on a deeper level, potentially revealing subtle inconsistencies and promoting clearer coding habits.
It's important to realize that the duck doesn't magically solve your problems; its value is in forcing a thorough, verbal examination of the code. By treating the process of debugging as a conversation, you naturally break down complex code into smaller, manageable chunks, fostering a more systematic and effective debugging process. It’s a simple yet effective approach that can ultimately improve your understanding of programming concepts and reduce frustration during debugging sessions.
Rubber Duck Debugging is a technique where programmers explain their code, line by line, to an inanimate object, often a rubber duck. It's a surprisingly effective approach that's been around since the early 2000s, gaining popularity through books like "The Pragmatic Programmer." The idea is that by vocalizing your code's logic and purpose, you're more likely to identify errors or inconsistencies in your thinking.
This method draws on a phenomenon known as the "protégé effect" where teaching or explaining something helps solidify one's own understanding. In this context, by explaining code to a rubber duck, programmers can essentially "teach" the code to the duck, thereby strengthening their grasp of its inner workings. It's almost like forcing yourself to think more deeply and meticulously about your code.
The duck acts as a sounding board, an audience that won't judge your logic or code. This can be a big help for programmers who sometimes hesitate to discuss their work with others for fear of criticism. Because it's a non-judgmental environment, programmers are free to explore their thoughts out loud, uncovering potential areas where their understanding of the code is incomplete or flawed.
In essence, the act of narrating the code helps to refine the programmer's mental model. By explaining the expected behavior of each part, you're forced to confront the assumptions and decisions you've made while writing the code. These explanations can sometimes reveal crucial errors or logical gaps that might otherwise slip by unnoticed. It's like a self-imposed code review, but one that's performed verbally.
Research suggests this method can be a real game-changer in terms of debugging effectiveness. By carefully stepping through the code, you can easily uncover bugs that might be tough to find with other debugging techniques. It's the careful articulation of the code, not the duck itself, that leads to success.
It's fascinating how taking the time to explain your code can sometimes lead to faster debugging than using sophisticated tools. It may feel counterintuitive, but the process of forcing yourself to vocalize each step of the code's logic helps identify and resolve issues more quickly.
It's also interesting that the Rubber Duck Method can serve as a bridge to better teamwork. If you're used to explaining your code aloud to a rubber duck, it becomes easier to transition those discussions into collaborative debugging sessions with other programmers. The habit of articulating your logic makes it easier for others to understand your thinking.
Beyond programming, the Rubber Duck Method finds use in fields like therapy and education. It seems that clarifying thoughts by speaking them out loud is universally helpful in solving problems and enhancing understanding.
The Rubber Duck Method is even more effective when paired with a structured approach to walking through your code. Following a defined pattern as you talk through the logic and purpose of each section helps you dissect complex issues into smaller, more manageable parts. This is particularly helpful when dealing with large, unwieldy codebases.
Finally, adopting this practice can boost your confidence in your problem-solving abilities. The more you practice explaining your code aloud, the better you become at identifying potential bugs and troubleshooting them. This practice turns debugging from an anxiety-ridden task into a more confident and organized process.
While the rubber duck may seem a bit silly at first, it is a powerful debugging technique that can improve the clarity of a programmer's thinking and help identify errors faster. It's an intriguing example of how simple ideas can sometimes produce significant gains in productivity.
More Posts from mm-ais.com: