7 Essential Coding Concepts Every Beginner Should Master in 2024
7 Essential Coding Concepts Every Beginner Should Master in 2024 - Variables and Data Types The Building Blocks of Programming
Variables and data types are the fundamental building blocks of any program. Think of variables as containers that hold information within a program. Each variable has a name, a value it stores, and a data type which defines what kind of information it can hold (like numbers, text, or true/false values). Importantly, the values stored in variables can be changed as the program runs, allowing for dynamic and flexible behavior.
Data types, in essence, define the characteristics of a variable's content and the operations that are permissible on that data. You have basic types, often called primitive data types, which act as the foundation for data handling. Things like whole numbers (integers), decimal numbers (floats), single characters, and Boolean values (true or false) are examples. Every language has its own collection of these, shaping the ways you can work with data.
Python, for instance, provides a wider range of data types including lists, tuples, dictionaries, and sets—each tailored for specific needs. Understanding and expertly using data types isn't just helpful, it's vital for efficiency and well-written code. It becomes crucial as your programs grow in complexity and scope. Essentially, getting comfortable with variables and data types is a must-have for any budding programmer. It's the springboard to more complex concepts and a foundation for creating functional and useful software.
1. Variables and data types act as the core building blocks of any program, enabling us to store and manage data effectively. The choices a developer makes in this area can significantly shape the efficiency and readability of their code.
2. Interestingly, different programming languages adopt distinct approaches to data type management. For instance, Python's flexibility with dynamic typing contrasts with the more rigid static typing found in C. This contrast has profound consequences for error detection and troubleshooting during development.
3. The idea of "null," a placeholder for the absence of a value, can be a tricky aspect of programming. If not handled carefully, it can lead to surprising and often frustrating errors, particularly the infamous "null reference exception."
4. The realm of data types goes beyond simple numbers and text. It extends to intricate structures like arrays, objects, and even custom-defined types, offering programmers a powerful toolkit to manipulate complex data.
5. The memory a program needs to store variables can vary considerably based on the data type. For example, an integer might occupy 4 bytes in a 32-bit application, while a floating-point number could require twice that amount. This relationship between data types and memory usage can have a noticeable impact on a program's performance, especially for large datasets or computationally intensive tasks.
6. Some languages provide type casting, a feature that allows developers to convert one data type to another. However, it's essential to understand that this conversion can sometimes lead to a loss of information or precision. Thus, type casting should be applied thoughtfully to avoid unforeseen errors.
7. The concept of variable scope is crucial in larger codebases. It determines where and for how long a variable is accessible within a program. This might be limited to a single function, a particular class, or even the entire application. Understanding scope is vital for preventing conflicts and errors that can crop up when variables are unexpectedly accessed or modified in different parts of a program.
8. Arrays offer a handy way to organize collections of related variables under a single name. But while they improve data management, they also introduce the potential for increased memory usage, especially when dealing with large datasets. This can put pressure on the system's resources, potentially slowing down performance.
9. The use of constants, values that remain fixed throughout the program, can substantially enhance both the readability and maintainability of code. By clearly labeling certain values as constants, developers signal that they should not be altered. This improves the understanding of the code for other developers who may need to work on the project later.
10. Many introductory programming environments simplify the complexities of variables and data types to help beginners get started. While this approach aids initial learning, it's crucial to recognize that these concepts are fundamental to writing efficient and reliable code in real-world development scenarios. Grasping these aspects becomes essential for creating programs that are both effective and free from unexpected errors.
7 Essential Coding Concepts Every Beginner Should Master in 2024 - Control Flow Statements Directing the Path of Execution
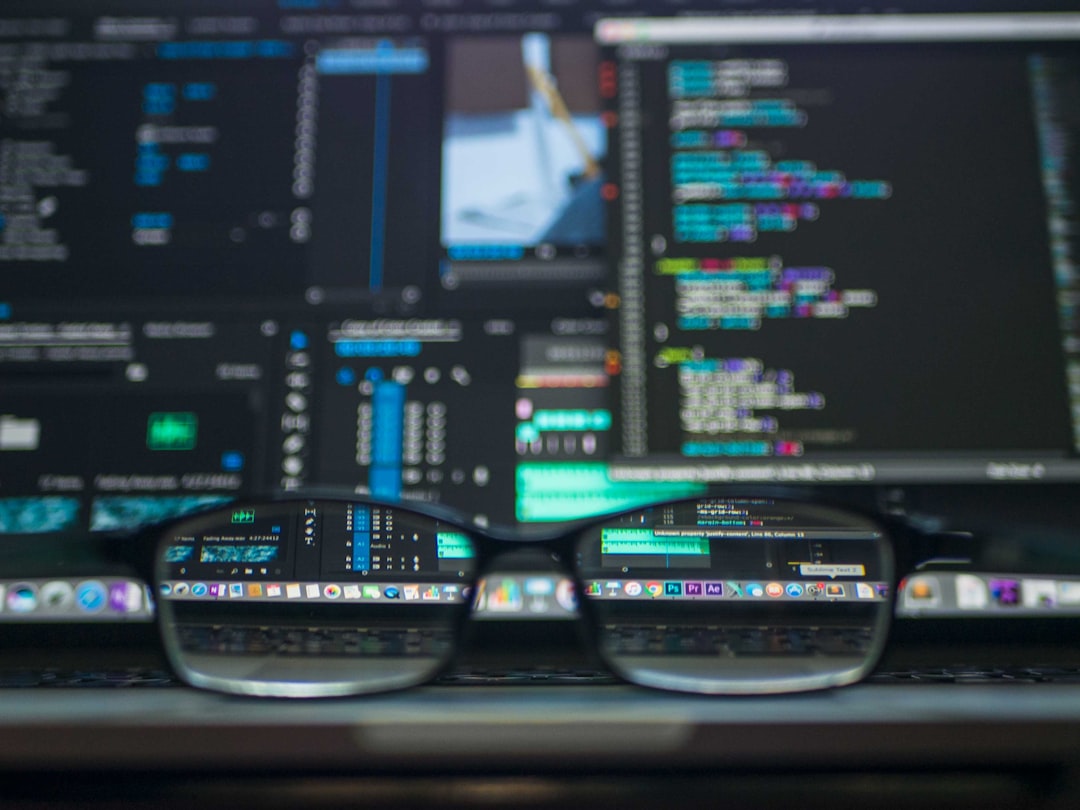
Control flow statements are the traffic signals of your code, guiding the execution path of your program. They are essential tools for creating programs that can respond dynamically to situations and user inputs. Without them, code would simply run line by line in a fixed order, lacking the ability to make choices or repeat actions.
Control flow statements empower programs to make decisions. This is achieved through conditional statements like `if` and `else`, allowing code to follow different paths based on whether certain conditions are met. They also manage repetition with loops like `for` and `while`, which execute blocks of code multiple times. Additionally, statements like `break` and `continue` allow for finer control over the flow by interrupting or skipping parts of the code.
Understanding control flow is a cornerstone of coding. It's what enables programmers to build complex, interactive, and adaptable software. If you're learning to code, becoming proficient in these control flow mechanisms will lay a strong foundation for writing effective and well-structured programs. It's a vital skill in creating applications that behave logically and efficiently in response to different circumstances and inputs.
Control flow statements are the conductors of a program, dictating the order in which instructions are executed. Without them, code would simply run in a straight line, severely limiting the ability to create adaptable and interactive applications. They empower programs to make decisions, repeat actions, and dynamically adjust their behavior based on conditions and user input.
The humble "if" statement, which enables conditional execution, can be surprisingly powerful when nested. However, overly complex nested structures can easily lead to a tangled mess known as "callback hell" or "pyramid of doom," making the code difficult to read and maintain. Switch statements offer a more elegant solution for handling multiple conditions based on a single variable, but they too can become unwieldy with convoluted logic.
Looping mechanisms like "for" and "while" are not interchangeable—choosing the right one can significantly impact performance and how variables behave within the loop. A subtle mistake can lead to an infinite loop, a dreaded situation where a program gets stuck repeating a task endlessly. This can freeze an application or even crash the system, emphasizing the need for careful planning and well-defined termination conditions.
Within loops, the "break" and "continue" statements can be handy for exiting or skipping iterations, but excessive use can make the code hard to follow. Modern programming languages have added intricate control flow features, like exception handling, for gracefully managing errors. However, if used without care, they can obfuscate the normal program flow and create hidden bugs.
The growing influence of functional programming paradigms has introduced new control flow patterns. Functions like map, filter, and reduce offer concise ways to handle collections but might seem less intuitive to those accustomed to traditional imperative approaches. Recursion, another form of control flow using self-referencing functions, can be remarkably elegant for certain tasks but can also cause stack overflow errors if not carefully crafted with proper base cases.
Fundamentally, grasping control flow is crucial because it impacts program performance and efficiency. Unoptimized control flow can lead to excessive resource consumption, potentially slowing down even the most potent hardware. Therefore, a deep understanding of these constructs is vital for crafting applications that are not only functional but also efficient and performant.
7 Essential Coding Concepts Every Beginner Should Master in 2024 - Functions and Modules Organizing Code for Reusability
Functions and modules are key to organizing code in a way that makes it reusable, which is vital for clean and efficient software. Think of functions as pre-written sets of instructions, like mini-programs, that can be called upon whenever needed. This cuts down on redundancy, as you don't have to rewrite the same code blocks over and over. This approach not only keeps code easier to manage—breaking down complex tasks into smaller, digestible pieces—but it also makes the overall code structure clearer.
Modules, especially in languages like Python, take this idea further. They package related functions, variables, and other components into separate files. This modularity leads to a more organized and reusable codebase. It's a strategy that encourages the isolation of specific tasks or features, making code easier to maintain and modify, especially as projects grow larger and more complex. If you're learning to code, understanding functions and modules is crucial. They form the foundation for creating cleaner, more efficient, and ultimately more sustainable software. It's a concept that will serve you well as you continue on your coding journey.
Functions and modules are like the organizational tools of code, primarily designed to promote reusability and make our code easier to manage. Imagine functions as pre-defined sets of instructions, like mini-programs within a larger program. They allow us to avoid writing the same code repeatedly, thus improving both efficiency and maintainability. To execute a function, we simply "call" it within our program using a specific syntax—which can vary between programming languages, but generally involves the function's name followed by parentheses.
Functions are also helpful for tackling complex problems by breaking them down into smaller, more digestible pieces. This approach leads to a better-structured and more organized codebase. Modules, especially popular in Python, take this organizational principle a step further by grouping related functions, variables, and classes into separate files. This modularity makes the entire codebase more manageable and promotes reusability—imagine a library of code components that you can pick and choose from.
A Python module, essentially, is just a file containing Python code identified by its .py extension. Modularization isolates functionalities, creating a kind of "divide and conquer" approach to code development. It becomes easier to manage, modify, and debug specific portions of a codebase, rather than having a single, giant interconnected block of code.
Mastering functions is crucial for clean and efficient code. They encapsulate a specific task, promoting a more readable and structured approach to code. Understanding how to define functions, call them, and pass arguments is essential for establishing solid coding practices.
Beyond functions, Object-Oriented Programming (OOP) introduces classes, providing another level of organization and reusability, working in conjunction with functions. OOP often complements modularity, further refining code structure. As our programs become larger and more complex, effective code organization into modules and packages is vital. It is considered a best practice to structure your code for improved maintainability and clarity.
While modular programming offers a plethora of benefits, there are some aspects to be mindful of. Recursion, a technique involving self-referencing functions, can be a powerful tool but needs to be handled carefully. An over-reliance on it can lead to performance issues, particularly in languages that don't optimize for recursion.
Furthermore, if functions are not meticulously designed with clear interfaces, they can introduce unintended consequences when reused in unforeseen ways. This emphasizes the importance of understanding the scope and implications of functions when integrating them into a larger program.
Ultimately, functions and modules are indispensable tools for developing well-structured and maintainable software. They enable a level of organization and reusability that leads to a more robust, understandable, and efficient development process. As we progress further into more complex coding scenarios, a thorough understanding of these concepts becomes essential to building software that is reliable and easy to adapt over time.
7 Essential Coding Concepts Every Beginner Should Master in 2024 - Object-Oriented Programming Principles Structuring Complex Systems
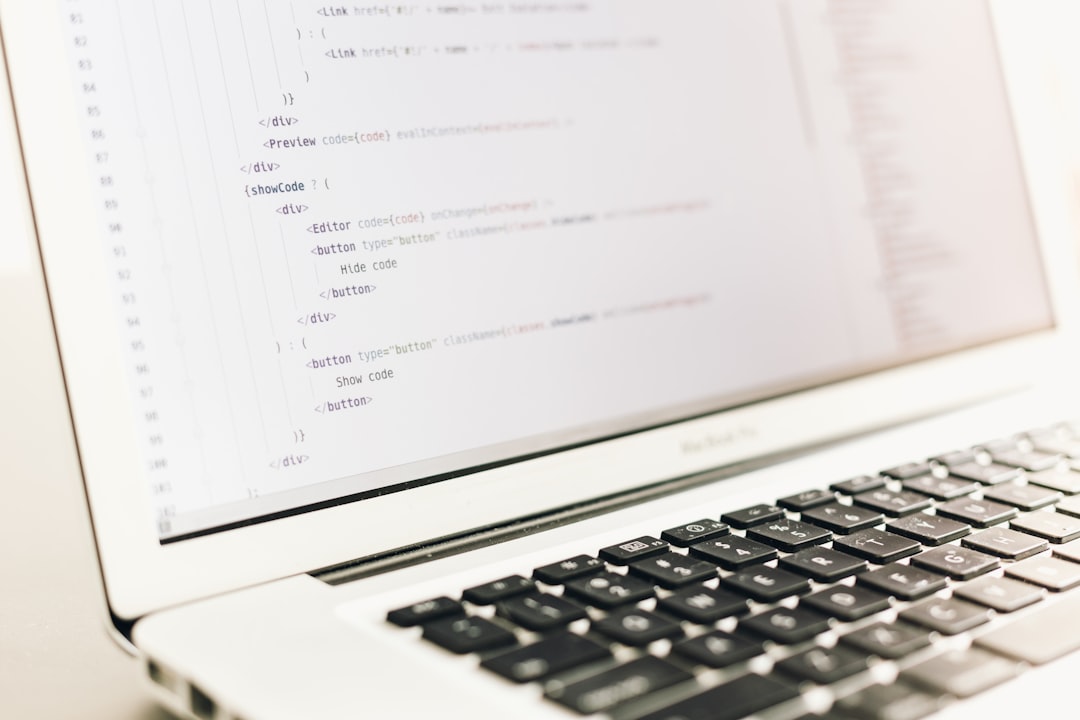
Object-Oriented Programming (OOP) provides a powerful way to tackle the intricate nature of modern software. It revolves around the idea of using "objects" to represent data and the actions performed on that data. This approach promotes a more organized and manageable structure compared to traditional methods.
Central to OOP are four key principles: abstraction, encapsulation, inheritance, and polymorphism. Abstraction simplifies complex systems by only showing the essential details and hiding the inner workings. Encapsulation ties data and its associated methods into a single unit, called a class, which helps to protect the data's integrity and control access. Inheritance allows new classes to build upon existing ones, reducing redundancy and building a clear relationship between different parts of the system. Finally, polymorphism gives different objects the ability to respond to the same method in their own unique way, which adds a lot of flexibility to how a system operates.
These principles help developers build systems that are more adaptable and easy to change. When starting out, understanding OOP is highly valuable. As programs become more complex, it's hard to manage the code without an organized structure, and OOP provides a framework for creating these structures. The concepts help make programs more understandable, easier to fix, and easier for multiple developers to work on together, all important aspects of modern software development.
Object-Oriented Programming (OOP) is a way to structure code using "objects" that combine data and actions. It's built on four fundamental ideas: abstraction, encapsulation, inheritance, and polymorphism, which are key to building software that's easy to change and expand.
Abstraction helps us focus on the important parts of an object and hide the complex stuff behind the scenes. This allows developers to work with objects without getting bogged down in intricate details, making the process more streamlined.
Encapsulation, on the other hand, bundles data and the operations that work on that data within a single unit, often called a "class." This safeguards the object's internal data and gives us fine-grained control over how that data is accessed. It's interesting to note that while this protects the data, it also makes code more maintainable because you can change an object's inner workings without affecting the code that uses it.
Inheritance provides a way for one class to inherit properties and actions from another. This promotes code reuse and establishes a hierarchical relationship between classes. While inheritance helps keep things organized, it can also lead to some pretty complex scenarios where changes to a parent class unexpectedly break its children—a problem called the "fragile base class" problem.
Polymorphism lets us treat different objects as if they were instances of a common parent class. This enables us to apply the same methods to various object types. It's worth noting that polymorphism is about more than just method overloading; it's also about designing systems with interfaces so different objects can provide similar functionalities in different ways, enhancing flexibility and adaptability.
OOP is extremely popular for building a wide array of applications, from basic desktop programs to complex enterprise software systems, mobile apps, and web platforms. Learning OOP is vital for beginners since it underpins many modern programming languages and frameworks. This approach gives code a clear structure, making it easier to understand and modify, especially when working in a team on a large project.
OOP fosters modularity, allowing components to be reused in different parts of a program or even in different projects. This concept significantly improves efficiency by reducing the need to rewrite the same code over and over. However, the modularity OOP promotes is often achieved through a communication process that leverages "message passing" rather than directly calling methods, which helps to keep the overall system loosely coupled and adaptable.
The idea that a class should ideally only have one reason to change is a guiding principle in OOP, often called the Single Responsibility Principle. Simple as it sounds, it forces clarity and focus in design, crucial for building reliable codebases as projects grow.
There's a strong argument to be made for "composition" as a better alternative to inheritance in some cases. Composition involves building a class by using references to other classes. This design leads to a more adaptable and maintainable system because it avoids some of the pitfalls of rigid class hierarchies, and it's generally more modular.
OOP designs often follow the Pareto principle, or the 80/20 rule, where 80% of the functionality comes from about 20% of the code. Understanding this can help developers prioritize their efforts and improve overall efficiency.
OOP also has a significant impact on testing. The modularity and concepts like encapsulation and abstraction make unit testing much easier. Testing each class individually is straightforward, leading to easier bug detection and more reliable software in the long run.
In essence, a solid understanding of OOP is indispensable for creating effective and maintainable software systems, especially as they scale and complexity increases. Understanding the potential pitfalls and tradeoffs is as crucial as understanding the principles themselves. The evolution of programming practices has revealed the importance of clear design and flexible architectures—OOP offers a set of powerful tools for this, but only if its principles are properly understood and applied.
7 Essential Coding Concepts Every Beginner Should Master in 2024 - Basic Algorithms and Problem-Solving Techniques Enhancing Logical Thinking
Basic Algorithms and Problem-Solving Techniques Enhancing Logical Thinking
Understanding basic algorithms and problem-solving techniques is crucial for developing strong logical thinking skills in coding. Algorithms, essentially step-by-step instructions for solving a problem, are fundamental for producing outputs from specific inputs. A good algorithm is characterized by its clarity, its ability to always produce a result, and its efficiency in using resources.
One of the key benefits of learning algorithms is the way they train programmers to approach complex tasks. Breaking down a problem into smaller parts, identifying recurring patterns, and applying structured thinking are all aspects of algorithmic thinking, skills that are highly valuable in programming. The practice of algorithm design encourages a programmer to reuse code components across different situations, thus reducing redundancy and improving the overall organization of the code.
It's important to practice applying these techniques. Working through coding challenges, even simple ones, can refine one's problem-solving skills and deepen the understanding of coding principles. This hands-on experience bridges the gap between theoretical knowledge and practical application.
Essentially, a solid grasp of algorithms and problem-solving strategies provides the foundation for any aspiring programmer. It builds a framework for logical thought and reasoning, which are essential skills for creating effective and efficient code as you advance your coding journey.
### Surprising Facts About Basic Algorithms and Problem-Solving Techniques Enhancing Logical Thinking
1. **Algorithm Efficiency's Nuances**: How efficient an algorithm is is often judged by how its execution time grows as the input gets bigger (time complexity) and how its memory needs change (space complexity). These measurements are essential because even with large datasets, a more efficient algorithm can dramatically reduce resource use.
2. **The Significance of Big O Notation**: Big O notation is a mathematical tool to describe the worst-case performance of an algorithm. It categorizes algorithms by their growth rates, providing a high-level understanding of how well a solution can scale as the input expands.
3. **Greedy vs. Dynamic Programming**: Greedy algorithms make the best choice available at each step, which can lead to good but not necessarily optimal overall results. In contrast, dynamic programming analyzes the entire problem and finds the best solutions by splitting it into smaller, overlapping subproblems, guaranteeing optimal outcomes.
4. **Exploring Problem-Solving Strategies**: There are different ways to tackle problems, and each might be better suited to specific types of issues. Techniques like backtracking, divide and conquer, and brute force provide diverse approaches, and choosing the right one significantly affects the clarity and performance of the solution.
5. **Recursion's Unexpected Costs**: Recursion, where a function calls itself, can simplify complex issues like traversing a tree structure within code. But it has hidden costs due to using extra memory for keeping track of multiple function calls, which, if not understood, can lead to stack overflow errors in languages that don't automatically optimize for recursion.
6. **The Value of Heuristics**: Heuristic techniques are often used when computing perfect solutions is impractical. They offer faster, approximate solutions, particularly useful in optimization problems like finding the shortest route or scheduling jobs where traditional approaches might be too slow.
7. **Unraveling P vs. NP**: The question of whether problems whose solutions can be quickly verified can also be quickly solved is a central unsolved problem in computer science. Known as the P vs. NP problem, it's fundamental to fields like cryptography and AI.
8. **Algorithm Variety's Impact**: Many core algorithms, like search and sorting algorithms (such as quicksort and mergesort), not only differ in their methods but also perform differently based on the characteristics of the data. For example, quicksort is often faster but can become very slow if the data is already sorted.
9. **Logical Thinking's Role in Coding**: Cultivating strong logical thinking abilities is crucial for aspiring programmers because the skill of breaking down complex challenges into simpler parts is key to the process of creating algorithms. Logical thinking is becoming more widely recognized as a fundamental skill in computational thinking.
10. **Algorithms in Everyday Life**: Beyond computer science, understanding algorithms and problem-solving approaches can improve reasoning and decision-making in everyday situations, such as optimizing travel routes, organizing tasks, and even handling finances.
These insights into algorithms and problem-solving not only help produce better code but also encourage critical thinking and analytical skills which are vital for programmers in the constantly changing technological landscape.
7 Essential Coding Concepts Every Beginner Should Master in 2024 - Version Control with Git Managing Code Collaboratively
In 2024, mastering Git—the most prevalent version control system—is essential for anyone involved in software development. Git empowers developers to meticulously track and manage changes made to their code, a crucial aspect of collaborative coding. The beauty of Git lies in its distributed nature, giving each developer a complete copy of the project's history. This distributed approach facilitates simultaneous development by multiple programmers without the worry of overwriting each other's work. Crucially, Git maintains a detailed record of each code modification, forming a historical trail that is invaluable for debugging, understanding project evolution, and resolving conflicts that can naturally arise during collaborative development. For beginners, getting a handle on core Git operations, including creating repositories, recording changes through "commits," and working with different versions ("branches") is paramount to being a productive member of a modern software development team. Without a solid grasp of these concepts, it's difficult to effectively navigate the complexities of shared codebases.
Git, the most widely used version control system (VCS), is essentially a tool for tracking changes to files over time. Developed in 2005 by Linus Torvalds, the creator of Linux, it addressed limitations of existing systems by adopting a decentralized approach. This has been transformative for software development, particularly in how developers collaborate on projects.
VCS like Git allow developers to save and keep track of every change made to their code. This means you can easily revert to previous versions if needed, a life-saver when bugs creep in. Furthermore, it allows developers to work collaboratively on a single project without accidentally overwriting each other’s efforts.
Getting started with Git is fairly straightforward. You can leverage platforms like GitHub or, alternatively, use the `git init` command in your terminal to initialize a Git repository. Notably, Git is a distributed VCS, meaning each developer on a team maintains a complete local copy of the repository. This decentralized design fosters better collaboration and resilience.
The beauty of Git lies in its ability to streamline collaborative development. Several team members can concurrently work on the same project without conflicting changes. Each alteration is recorded, providing a detailed history of modifications. This history is invaluable for developers as they review and debug the codebase.
For beginners, some key concepts in Git are essential to grasp: understanding what repositories are, how commits work, the role of branches, merging branches, and the occasional challenge of conflict resolution. It’s a bit of a learning curve but a worthwhile one.
Modern software development heavily relies on version control. It's the backbone of project management, promoting a methodical approach to maintaining and organizing code. Git's decentralized model is particularly useful, benefiting both lone developers and large teams across diverse geographic locations. This allows for great flexibility in how work is organized.
In the world of 2024, mastering Git isn’t just a nice-to-have, it’s essential. Being fluent in Git is now recognized as a cornerstone skill for software engineers. It underpins your ability to effectively manage code and work with others, a vital component of developing modern software.
Git's branching capability is powerful, allowing developers to work on new features or fixes without disturbing the main codebase. This promotes experimentation while preserving stability. It’s akin to having multiple “draft” versions of a project.
A particularly interesting facet of Git is its commit history. Every commit acts like a snapshot of the project at that specific point. This history is a powerful debugging tool, allowing us to trace bugs back to their origins, significantly speeding up troubleshooting.
Merging in Git is an important tool for integrating work from different branches. While the concept of merging is straightforward, it can sometimes lead to conflicts when changes in one branch conflict with changes in another. This can seem intimidating at first but, understanding how to manage those conflicts is fundamental for collaboration.
Git's distributed nature means that every developer has a complete copy of the project. This approach offers several advantages, including redundancy, offline access to your work (important for those who work remotely), and increased resilience in case something happens to the main server.
The use of Git can lead to an improved code quality by encouraging consistent commit practices and informative commit messages. This consistent, well-documented history enhances collaboration and promotes a culture of code review, helping improve software quality in the long run.
The speed of iteration also accelerates with Git. Using lightweight branches makes experimenting with new features a breeze. You can quickly try out new ideas and, if they don't work out, just discard the branch without damaging the main codebase. This creates a more innovative development process.
Git offers a ‘stash’ function to save changes that you don’t want to commit yet. This can be particularly useful if you need to switch branches suddenly or want to quickly jump to a different task without disrupting your current progress.
The open-source software world is inextricably tied to Git. Platforms like GitHub have revolutionized the process of open-source contributions. Developers worldwide can collaborate on projects easily thanks to Git, leading to a remarkably dynamic environment for developing software.
While Git’s basic features are relatively easy to learn, truly mastering its capabilities requires dedication. Understanding more advanced features like rebasing, cherry-picking, and interactive staging can take time and effort. However, these advanced features expand your repertoire, enabling you to tackle highly complex projects with greater ease.
In conclusion, Git has become an indispensable part of modern software development. Learning it is crucial for anyone who wants to code professionally in 2024. It's not just about managing code, it’s about effectively collaborating, experimenting, and producing high-quality software in a rapidly evolving world.
7 Essential Coding Concepts Every Beginner Should Master in 2024 - Debugging and Error Handling Ensuring Robust Code
Debugging and error handling are essential skills for anyone who wants to build software that's reliable and works as intended. New programmers should learn how to manage errors gracefully, using techniques like try-catch blocks to handle exceptions and prevent crashes when things go wrong. It's also important to understand the different types of errors that can pop up: compile-time errors, which are caught before the program runs, runtime errors that happen during execution, and logical errors, where the code doesn't do what it's supposed to.
Modern tools like integrated development environments (IDEs) can be a great help. Many have built-in debuggers that let you pause the code at specific points, inspect variables, and step through the program line by line to track down the source of problems. A crucial aspect of debugging is developing a flexible mindset—expect errors, be ready to experiment to find what's wrong, and learn from each mistake you encounter. These skills of identifying, understanding, and fixing errors are key to becoming a successful developer. Learning to debug effectively ensures that your programs are more stable, easier to maintain, and produce the correct output, making your code much more trustworthy.
Debugging and error handling are essential for building reliable and robust software. While we often focus on the initial stages of coding, the reality is that errors are an inevitable part of the process. Understanding how to identify and resolve these issues is fundamental for any aspiring programmer.
One of the most common causes of errors is human mistakes. This emphasizes the need for careful coding practices and rigorous testing to minimize these issues. The cost of fixing bugs can vary widely depending on when they're discovered. Fixing a bug in the early stages can be significantly cheaper than dealing with it after the software has been released, making early debugging crucial.
Interestingly, effectively handling errors can sometimes even improve a program's performance. For example, if we anticipate potential problems and build checks into our code, it can sometimes reduce the need for repeated error checks, making the program run more efficiently. A subtle challenge in error handling arises when we encounter "silent failures," situations where a program continues to operate but delivers incorrect results without obvious warning signs. Robust logging mechanisms are vital in such cases to detect these problems.
Peer review and code reviews have a measurable impact on code quality. Reviews can catch many errors before they end up in the final software, reducing the burden on debugging during later stages. The debugging tools we have today are quite advanced, including specialized tools like debuggers, linters, and code profilers. Despite this, many developers still resort to manual debugging, which can be less efficient.
Exception handling is a powerful tool that gives us a better way to separate normal program flow from errors. This improves the readability of code. However, it's worth noting that poorly designed exception handling can actually make the code more difficult to maintain if not carefully considered. Interestingly, a sizable proportion of errors in software often stems from just a small number of code modules. Focusing debugging efforts on these high-error areas can yield disproportionately positive results.
Testing plays a vital role. Modern approaches like behavior-driven development (BDD) go beyond conventional testing by placing the user's perspective at the heart of the tests. This is incredibly useful in catching errors that might otherwise be missed and also improves communication within development teams.
Debugging can be a frustrating experience, so it's important to recognize that the psychological aspects of debugging can significantly affect the process. Sometimes, simply explaining the problem to someone else or even an inanimate object (known as rubber duck debugging) can trigger new insights and alleviate stress.
Ultimately, robust code involves not just writing the initial code effectively but also mastering the skills to identify and fix errors efficiently. Understanding the intricacies of debugging and error handling is vital for building truly reliable software and is a skill that will become even more important as we move forward.
More Posts from :