5 Native JavaScript Alternatives to Common jQuery Functions in 2024
5 Native JavaScript Alternatives to Common jQuery Functions in 2024 - Document Selection Made Easy with querySelector
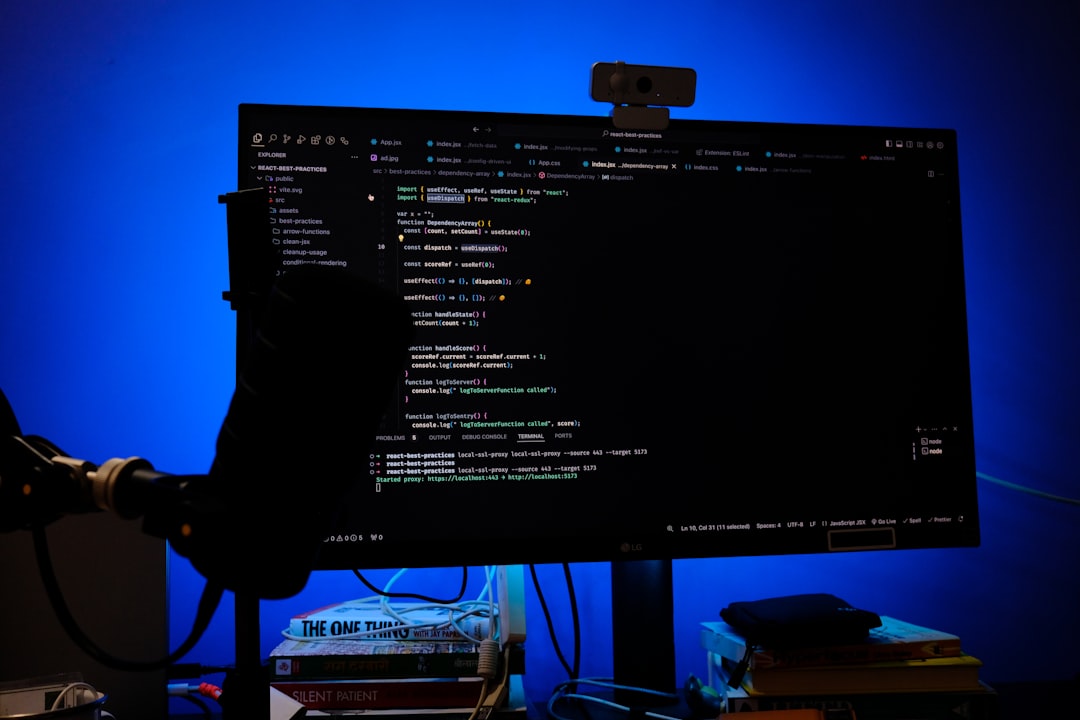
JavaScript's `querySelector` and `querySelectorAll` methods have become fundamental for working with the Document Object Model (DOM). Leveraging CSS selector syntax, `querySelector` efficiently retrieves the first matching element, while `querySelectorAll` captures all matching elements within the document. The latter returns a NodeList, which is not a standard JavaScript array. This means you need to employ methods like `forEach` if you want to loop through its contents, which can be a minor hurdle for developers accustomed to jQuery's more seamless array-like handling of results. While JavaScript's native capabilities have become more robust and have filled in many areas previously dominated by jQuery, understanding the subtleties of how these selection methods operate is key. Notably, it's important to anticipate situations where no matching elements are found, as `querySelector` returns `null`, while `querySelectorAll` yields an empty NodeList. Additionally, improper CSS selectors can lead to `SYNTAXERR` exceptions, highlighting the importance of accurate syntax. As the JavaScript landscape continues to evolve, developers can continue their journey away from jQuery with greater confidence, provided they are aware of these particular nuances when managing DOM elements.
JavaScript's `querySelector` and `querySelectorAll` methods offer a streamlined way to interact with the Document Object Model (DOM) by using CSS selectors. This means we can apply our knowledge of CSS styling to pick out specific HTML elements, simplifying our code structure.
Interestingly, unlike jQuery's `$()` which wraps the selected element in a jQuery object, `querySelector` directly returns the actual DOM element. This direct access can boost performance, especially in situations where we're frequently manipulating the DOM.
Moreover, `querySelector` isn't restricted to simple selections. It allows for sophisticated queries with features like pseudo-classes (e.g., `:nth-child()`), providing more flexibility for pinpointing elements within our web pages.
However, there's a key difference between jQuery's approach and `querySelectorAll`'s output. `querySelectorAll` returns a NodeList, which is a static collection. This means the collection won't update automatically if the DOM changes, which might not always be intuitive for developers used to jQuery's dynamic collections.
Both `querySelector` and `querySelectorAll` are efficient, often reducing the need for multiple method chains. We can achieve what traditionally required several steps with a single line of code.
It's crucial to note that `querySelector` returns the first matching element, whereas `querySelectorAll` provides a list of all matches. Understanding this difference is important to avoid any surprises when working with multiple elements.
Browser support for these methods is now extensive, with widespread adoption across modern browsers. This consistent support makes them a reliable choice for DOM manipulation in current web projects.
`querySelector` also allows us to leverage custom attributes like data attributes when targeting elements, facilitating scenarios where components utilize dynamic data for selection.
The `:not()` pseudo-class further enhances our capabilities by allowing exclusions within our selections. This feature can refine our code by reducing the need for filtering logic, leading to cleaner and more efficient JavaScript.
While `querySelector` is a powerful tool, relying on it excessively for many selections within complex pages can introduce performance issues. We should strike a balance and use it selectively to ensure fast manipulation and access to elements.
5 Native JavaScript Alternatives to Common jQuery Functions in 2024 - Event Handling Without jQuery's on Method
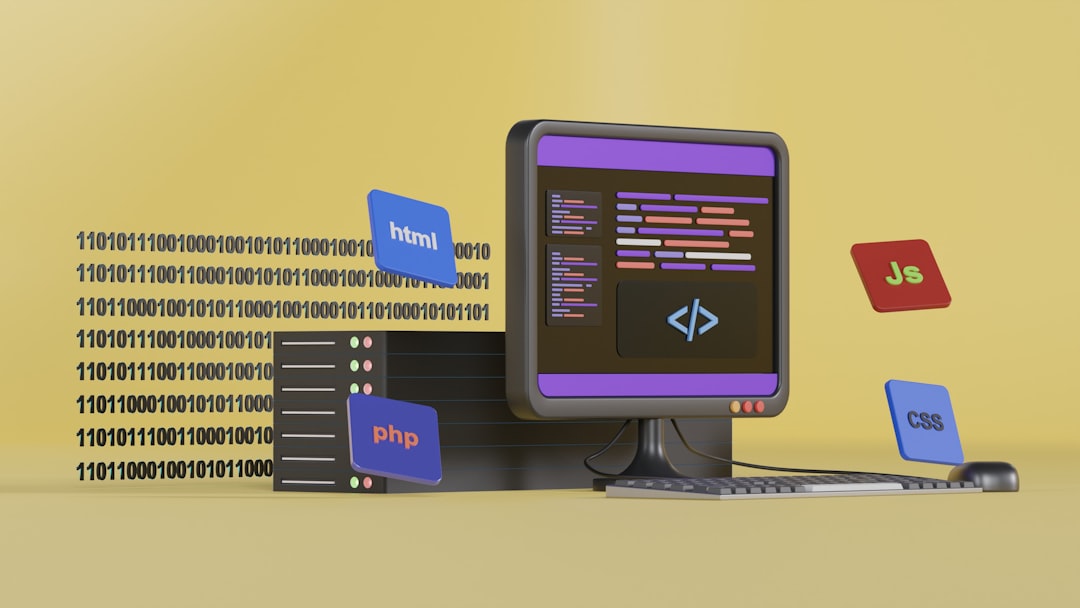
Moving away from jQuery's `on` method for event handling isn't difficult with native JavaScript. The `addEventListener` method provides a core alternative, allowing you to attach event listeners directly to elements. This creates a more flexible and modular approach to interactions. It's also worth noting that `DOMContentLoaded` in JavaScript works similarly to jQuery's `$(document).ready()`, ensuring your scripts execute after the HTML document is fully loaded, which is a basic necessity. Furthermore, event delegation is readily achievable in plain JavaScript, allowing you to manage events for dynamically added elements by using a parent element as the listener. Lastly, understanding how events propagate – including bubbling and capturing – is important to effectively control how events trigger actions across elements within a page. By utilizing these built-in features, you can reduce your reliance on jQuery while maintaining or even improving the responsiveness of your web applications. While jQuery's syntax might seem simpler at first glance, learning these native methods provides more direct control and often, better performance in the long run. It's a useful skill to develop as JavaScript continues to evolve.
JavaScript's `addEventListener` method offers a native way to handle events, providing a similar functionality to jQuery's `.on()` method. While `addEventListener` might seem less concise initially, it allows for attaching multiple event types to a single element without the need for jQuery's extra layers, leading to code that's potentially cleaner and easier to maintain. However, it's important to understand the nuances of how `addEventListener` works, particularly in the context of event capturing and bubbling. Controlling whether an event handler is executed during the capturing or bubbling phase allows for more precise event handling than might be readily apparent with jQuery's `.on()` method.
Managing event listeners directly can also lead to performance considerations that jQuery's `.off()` method conveniently handles. Carefully removing unused listeners is crucial to avoid memory leaks, especially in complex applications, which isn't always intuitive. Unlike jQuery, which often handles events in a more universal manner, JavaScript favors more type-specific methods for handling individual event types like clicks, key presses, and mouse movements. This might seem like extra work, but it potentially helps in avoiding unintended interactions between different event types.
The use of named functions with `addEventListener` rather than anonymous functions improves the organization of code by clearly defining each listener's function, which makes removal later much easier. This could be seen as more robust practice for preventing unintentional side effects. Furthermore, the `options` parameter of `addEventListener` gives access to features like specifying whether an event is passive or handled in the capturing phase. For example, you can optimize scrolling behavior for touch and wheel events.
Event delegation remains a powerful technique in native JavaScript. It can be refined using the `closest()` method in combination with `addEventListener()`, allowing us to efficiently handle events for dynamically added elements within a parent without adding individual listeners to each child. This leads to more streamlined and efficient code, especially when dealing with lists or large datasets. When events change in dynamic lists, delegating events to a single parent can be very effective in limiting the number of handlers required, thus significantly improving performance.
Removing individual event listeners with `removeEventListener()` is straightforward, although it requires remembering to pass the exact same reference to the listener function used when it was originally added. This, compared to jQuery, might add another mental step for the developer in tracking which function is attached to each listener. Overall, event handling in native JavaScript using `addEventListener` provides a solid foundation for managing events without external libraries. But it does require a bit more care in certain aspects. Understanding these unique aspects and taking proactive steps to manage performance and code organization is a necessary step in this shift away from jQuery.
5 Native JavaScript Alternatives to Common jQuery Functions in 2024 - AJAX Requests Using the Fetch API
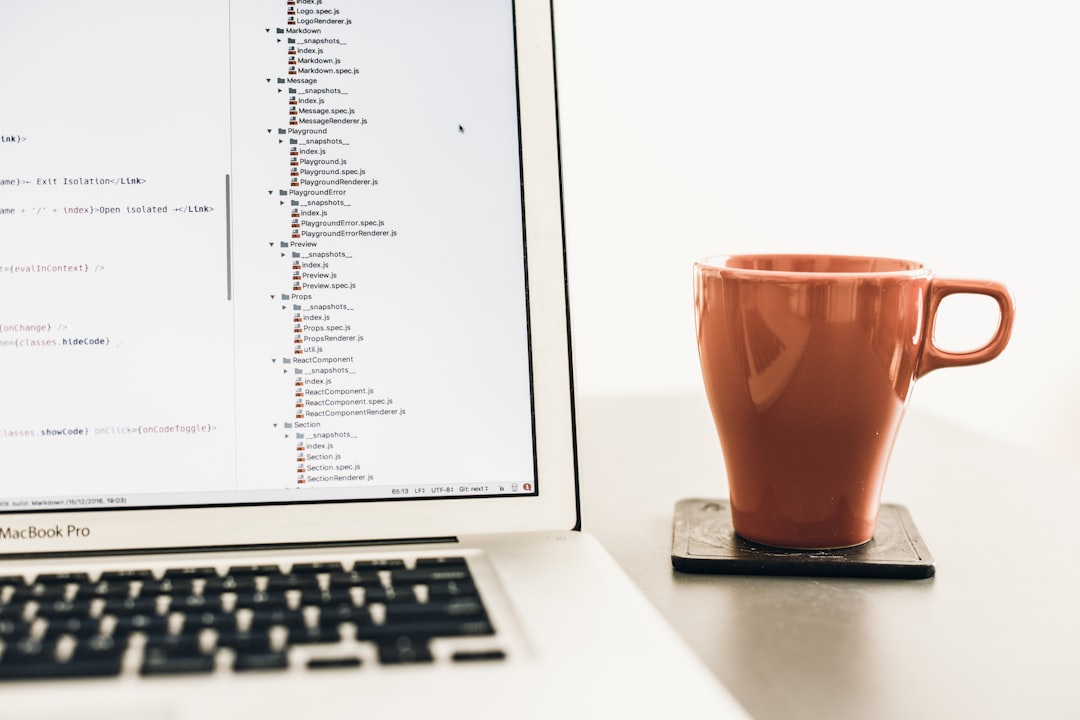
The Fetch API offers a modern approach to AJAX requests, replacing the older XMLHttpRequest method. It simplifies asynchronous operations by utilizing Promises, making error handling and code organization more intuitive with techniques like `async/await`. Compared to jQuery's AJAX functions, which often hide complexities, the Fetch API necessitates a more hands-on approach to request details, like converting data to strings for payloads and setting headers. This explicit handling can lead to a better understanding of how requests work and aligns with current JavaScript development practices. While the Fetch API provides significant improvements, it's crucial for developers to understand its limitations and be aware of scenarios where older methods might still be useful. There are some things to watch out for when working with the Fetch API that are not always so easy, like making sure data sent in the request is correctly formatted, and dealing with responses that are not JSON based. It does take a bit of learning.
The `fetch` API presents a contemporary approach to AJAX requests, offering a more streamlined and promise-based alternative to the older `XMLHttpRequest`. By returning a Promise, it naturally handles asynchronous operations, avoiding the intricate callback structures common in earlier JavaScript. This can lead to cleaner and more readable code, especially for developers who find themselves dealing with complex chains of asynchronous tasks. Interestingly, unlike its predecessor, `fetch` provides support for streaming requests and responses. This feature is especially useful when interacting with large datasets or in real-time applications, allowing for more efficient management of the data flow.
However, `fetch`'s error handling has its own quirks. It only rejects the promise in cases of network failures or situations that block a request from completing. HTTP error responses like 404s or 500s don't trigger automatic rejection; instead, developers must examine the `response.ok` property, which adds a layer of complexity to error management. Another aspect that developers need to be aware of is the default behavior of `fetch` when it comes to credentials (like cookies). By default, it does not include any credentials, which is a security measure designed to mitigate risks in cross-origin requests. However, this can be problematic if you need to share authentication or session data in your applications. It's crucial to use the `credentials` option if you want your requests to include credentials, which adds another step to the process compared to something like jQuery's `$.ajax`.
Following the security principle of least privilege, `fetch` embraces the same-origin policy. This restricts cross-origin requests unless specifically allowed via server-side CORS (Cross-Origin Resource Sharing) headers. While enhancing web security, it can also add some complexity to workflows when trying to interact with servers on different domains, leading to potential integration challenges that jQuery's `$.ajax()` might handle more automatically. When dealing with JSON, `fetch` offers an easy solution through the `response.json()` method, which gracefully parses responses. This significantly simplifies the process compared to manual parsing, as was necessary with `XMLHttpRequest`.
Further enhancing `fetch`'s capabilities is the ability to integrate `AbortController`. This feature enables the cancellation of requests that are in progress, which can be valuable when users interact dynamically with the application. This is a handy feature that is generally missing in jQuery's AJAX methods. The `fetch` API also supports the direct configuration of request methods, headers, and body content, streamlining the construction of even elaborate requests. Additionally, the `fetch` API supports operations on the Request and Response objects themselves. This provides for sophisticated use cases, such as cloning a request to use it again with different responses.
Despite its strengths, `fetch` comes with a notable omission: inherent timeout functionality is missing. To handle scenarios where a server is slow to respond, developers need to implement custom timeout logic using Promises, potentially increasing the complexity of what's otherwise a user-friendly API. This lack of built-in timeouts is a design choice that can lead to some added work if you aren't anticipating it. The `fetch` API, while a welcome upgrade in AJAX functionality, does come with a few interesting considerations that are worth keeping in mind. As JavaScript's ecosystem continues to evolve, it's becoming increasingly important for developers to understand the specific features of each built-in tool.
5 Native JavaScript Alternatives to Common jQuery Functions in 2024 - DOM Manipulation Through Native JavaScript Methods
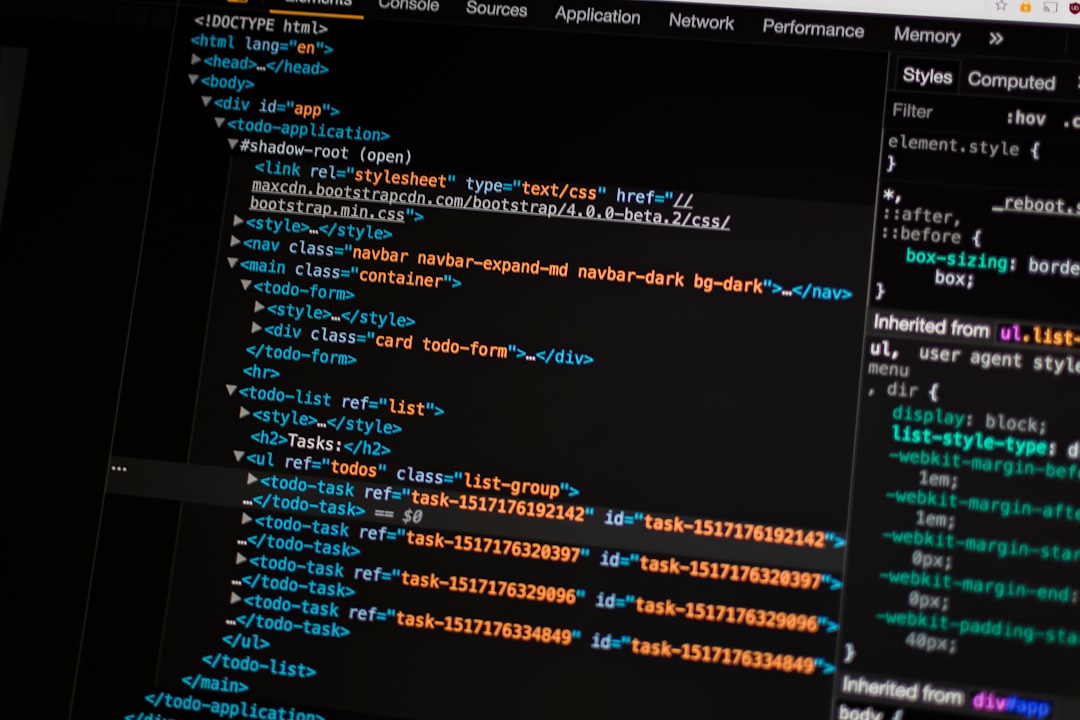
The way we manipulate the Document Object Model (DOM) in web development is changing, driven by advancements in native JavaScript. While jQuery once held a prominent position, newer methods like `querySelector`, `classList`, and `addEventListener` have become the preferred options for tasks such as selecting elements, managing classes, and handling events. This shift towards "vanilla" JavaScript often leads to more efficient applications with fewer dependencies. It's a move that requires understanding how the DOM works at a deeper level. As the community moves away from jQuery, developers need to learn the intricacies of these native methods for building high-quality, maintainable web applications. Fortunately, most modern browsers support these methods well, making them a dependable choice for current projects. While jQuery's ease of use was alluring, having a deeper grasp of the DOM itself brings the ability to craft cleaner and often faster applications that are less reliant on external libraries.
While jQuery has historically been a go-to for simplifying DOM manipulation, the performance benefits of using native JavaScript methods like `querySelector` and `addEventListener` are becoming more apparent, especially in performance-sensitive applications. This shift away from jQuery's reliance on object wrapping can lead to measurable gains, but also requires a closer understanding of JavaScript's core features.
One key distinction between jQuery and native methods lies in how they handle collections of DOM elements. jQuery offers dynamic collections, automatically updating as the DOM changes, whereas `querySelectorAll` returns a static NodeList. This difference can be problematic if you expect the collection to be updated automatically.
Furthermore, the use of CSS selectors with `querySelector` creates a dependence on CSS syntax. This can result in elegant code when used effectively, but errors or misuse can lead to issues. Developers have to be acutely aware of CSS selector rules, as this forms the basis of how elements are targeted.
Native event handling with `addEventListener` offers a high level of granularity in controlling the propagation of events through the capturing and bubbling phases. This nuanced control over event flow provides developers with greater flexibility in controlling how and when events trigger actions. But it does require developers to be mindful of this behavior within the structure of their applications.
The ability to manage events directly also comes with the responsibility of memory management. In contrast to jQuery's more automated approach to listener removal, native JavaScript's `removeEventListener` function demands that you carefully remove listeners to prevent potential memory leaks. While this can be seen as a slight burden initially, it ultimately encourages a more disciplined coding style that could prevent issues in more complex applications.
Moving to the Fetch API, we encounter some changes from jQuery's AJAX functions. Specifically, error handling is more explicit and less automatic. Fetch only rejects a request promise on network failures and blocking errors. HTTP error responses like 404s or 500s don't trigger automatic rejection; they must be checked in the `response.ok` property. This adds an extra step to the developer workflow but, in exchange, provides a clearer picture of the requests and responses at play.
The Fetch API also supports streaming requests and responses, a feature that older jQuery AJAX methods did not natively handle. This is valuable for scenarios involving very large data sets or real-time updates as it facilitates more efficient management of the flow of data.
Interestingly, the Fetch API currently doesn't include built-in timeout logic, which jQuery's AJAX methods offered. This necessitates developers to add their own timeout functionality when needed, which increases complexity and requires careful attention to the overall request flow.
Lastly, Fetch's adherence to the same-origin policy enforces a strict approach to cross-origin resource sharing (CORS) that developers need to be aware of. While security is the key driving factor here, it can be a significant shift for those used to jQuery's automation of these interactions. Developers need to manually configure CORS headers to properly interact with servers on different domains.
Moreover, the Fetch API necessitates manual parsing for certain non-JSON responses. jQuery's AJAX methods handle many different response types gracefully, but Fetch only automatically handles JSON; other types must be specifically parsed.
In summary, transitioning from jQuery to native JavaScript necessitates a shift in thinking about how to manage DOM elements, events, and network requests. While it offers a deeper understanding of JavaScript and control over your application's behavior, it requires a disciplined approach to managing potential performance and error handling concerns. As JavaScript continues to evolve and provide native solutions for many areas previously served by libraries like jQuery, the shift in perspective and attention to detail is becoming increasingly important for developers building reliable and efficient web applications.
More Posts from mm-ais.com: